vue 根据ref强制刷新
时间: 2023-07-05 20:10:43 浏览: 117
在 Vue 中,可以通过 `$forceUpdate` 方法强制更新组件,但是它只会更新组件的渲染,不会更新组件的 DOM 。
如果你想要根据 `ref` 强制刷新一个组件,可以先通过 `$refs` 获取到该组件的实例,然后再调用其 `$forceUpdate` 方法。例如:
```html
<template>
<div>
<my-component ref="myComponent"></my-component>
<button @click="forceUpdateMyComponent">强制刷新</button>
</div>
</template>
<script>
export default {
methods: {
forceUpdateMyComponent() {
this.$refs.myComponent.$forceUpdate()
}
}
}
</script>
```
以上代码中,我们在模板中定义了一个名为 `myComponent` 的组件,并使用 `ref` 属性给它起了一个别名。然后在按钮的点击事件中,我们通过 `$refs` 获取到了该组件的实例,并调用了其 `$forceUpdate` 方法,来强制更新该组件的渲染。
相关问题
vue3中强制刷新页面
vue3中可以使用`$route`对象的`params`属性来实现强制刷新页面。具体方法如下:
1. 首先,在需要强制刷新的组件中,通过`import { ref, watch } from 'vue'`导入`ref`和`watch`函数。
2. 在组件的`setup`函数中创建一个响应式引用,用于监听`$route`对象的`params`属性的变化。例如:`const refresh = ref(false)`
3. 使用`watch`函数监听`$route.params`,并在回调函数中将`refresh`的值置为`true`。例如:
```javascript
watch(() => $route.params, () => {
refresh.value = true
})
```
4. 在组件的模板中,使用`v-if`指令根据`refresh`的值来决定是否重新渲染组件。例如:
```html
<template>
<div v-if="refresh">
<!-- 组件内容 -->
</div>
</template>
```
5. 当需要强制刷新页面时,只需修改`$route.params`的值即可。
6. 当`$route.params`的值发生变化时,组件会重新渲染,从而实现强制刷新页面的效果。
vue中强制刷新其他指定组件完整代码
在 Vue 中强制刷新其他指定组件可以使用 `this.$forceUpdate()` 方法。下面是一个简单的示例代码:
```
<template>
<div>
<button @click="refreshComponentB">刷新组件B</button>
<component-a />
<component-b ref="componentB" />
</div>
</template>
<script>
import ComponentA from './ComponentA.vue'
import ComponentB from './ComponentB.vue'
export default {
components: {
ComponentA,
ComponentB
},
methods: {
refreshComponentB() {
this.$refs.componentB.$forceUpdate()
}
}
}
</script>
```
在上面的代码中,我们通过 `ref` 属性给组件B起了一个名称,然后在 `refreshComponentB` 方法中通过 `$refs` 来获取该组件实例,并调用 `$forceUpdate` 方法来强制刷新该组件。需要注意的是,只有在组件的数据发生变化时,组件才会重新渲染,因此我们需要确保在调用 `$forceUpdate` 方法之前已经修改了该组件的数据。
阅读全文
相关推荐
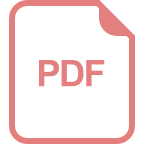
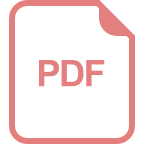
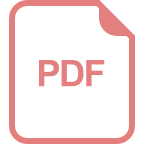













