使用C语言读入某字符串,统计此字符串中(不含'\O),数字、小写字母、大写字母、空格、其他字符分别有多少个?例:输入abc,DEFG 12345回车输出5,3,4,1,1
时间: 2024-02-07 17:02:13 浏览: 73
好的,可以使用C语言的字符处理函数来完成这个任务。以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int digits = 0, lowercase = 0, uppercase = 0, space = 0, others = 0;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) {
digits++;
} else if (islower(str[i])) {
lowercase++;
} else if (isupper(str[i])) {
uppercase++;
} else if (isspace(str[i])) {
space++;
} else {
others++;
}
}
printf("数字:%d\n", digits);
printf("小写字母:%d\n", lowercase);
printf("大写字母:%d\n", uppercase);
printf("空格:%d\n", space);
printf("其他字符:%d\n", others);
return 0;
}
```
解释一下代码:
1. 首先定义了一个`str`数组用于存储输入的字符串,以及五个变量用于分别记录数字、小写字母、大写字母、空格、其他字符的数量。
2. 使用`fgets()`函数从标准输入中读入一个字符串,存储到`str`数组中。
3. 使用`for`循环遍历`str`数组中的每个字符,如果是数字、小写字母、大写字母、空格或其他字符,则分别对应的计数器加一。
4. 最后输出各个计数器的值即可。
需要注意的是,上述代码中使用了`ctype.h`头文件中的一些字符处理函数,如`isdigit()`、`islower()`、`isupper()`和`isspace()`等,这些函数可以判断一个字符是否是数字、小写字母、大写字母或空格。如果不熟悉这些函数的使用,可以查看相关的C语言教程。
阅读全文
相关推荐
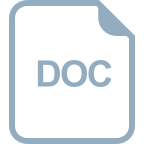
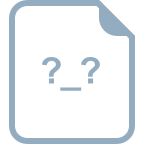
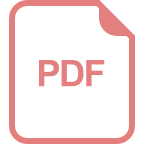















