linux做三个本地用户登录,进行密码验证后操作目录转至用户家目录
时间: 2024-10-11 16:08:01 浏览: 23
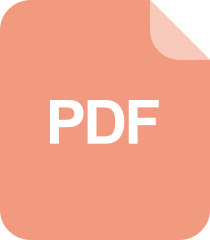
linux远程登录ssh免密码配置方法

在Linux环境下使用C语言创建一个简单的程序,让用户通过输入用户名和密码进行身份验证并切换到各自的家目录,你可以使用`getpass`库来隐藏用户输入的密码,同时利用`pam_access`模块进行权限检查。以下是一个基本的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <pwd.h>
#include <grp.h>
#include <getopt.h>
#include <sys/types.h>
#include <security/pam_appl.h>
#define PASSWORD_FILE "/path/to/passwords.txt" // 实际应用中应加密存储
// 用户数据结构
typedef struct {
char username[64];
char password[64];
} User;
void authenticate(User *user) {
FILE *password_file = fopen(PASSWORD_FILE, "r");
if (password_file == NULL) {
perror("Failed to open password file");
return;
}
char input_password[64];
fgets(input_password, sizeof(input_password), stdin);
input_password[strcspn(input_password, "\n")] = '\0'; // 移除换行符
char stored_password[64];
fscanf(password_file, "%s\n", stored_password);
if(strcmp(stored_password, user->password) == 0) {
printf("Authentication successful.\n");
// 根据username获取home路径
struct passwd *passwd = getpwnam(user->username);
if (passwd != NULL) {
chdir(passwd->pw_dir); // 切换到用户家目录
} else {
perror("Failed to find user home directory");
}
} else {
printf("Incorrect password.\n");
}
fclose(password_file);
}
int main(int argc, char *argv[]) {
const char* usage = "Usage: %s [-u username] [-p password]\n";
User user;
while ((argc > 1) && (argv[1][0] == '-')) {
switch (argv[1][1]) {
case 'u':
strncpy(user.username, argv[2], sizeof(user.username));
break;
case 'p':
strncpy(user.password, argv[3], sizeof(user.password));
break;
default:
fprintf(stderr, usage, argv[0]);
exit(EXIT_FAILURE);
}
argc -= 2;
argv += 2;
}
if (argc != 0) {
fprintf(stderr, usage, argv[0]);
exit(EXIT_FAILURE);
}
if (!user.username || !user.password) {
printf("Please provide both username (-p).\n");
return EXIT_FAILURE;
}
authenticate(&user);
return 0;
}
```
在这个示例中,你需要一个包含用户名和对应明文密码的文件(不推荐),并且密码文件需要妥善保管,因为它是明文的。
运行这个程序时,可以通过命令行提供用户名和密码,例如:
```sh
./my_program -u user1 -p password1
```
阅读全文
相关推荐
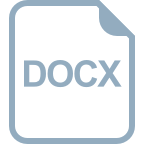
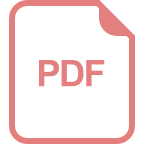

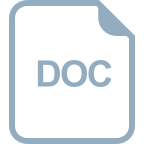
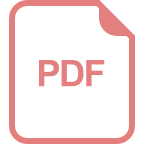
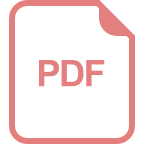
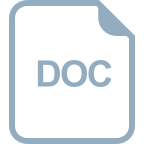
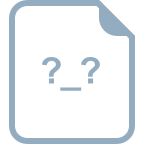
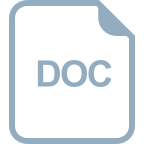
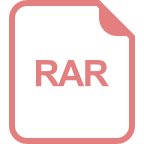
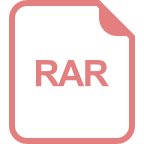
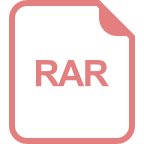
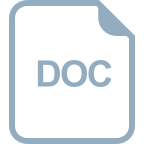
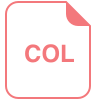
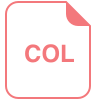
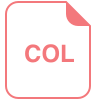
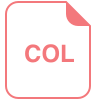
