c语言进栈出栈完整代码
时间: 2023-12-12 09:34:42 浏览: 78
以下是C语言实现栈的完整代码,包括初始化栈、扩容、入栈、出栈、获取栈顶元素、获取栈中有效元素个数、检测栈是否为空和销毁栈等操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
#define STACK_SIZE 4 // 初始容量
typedef int STDataType;
typedef struct Stack {
STDataType* base; // 数组
STDataType* top; // 栈顶指针
int stackSize; // 容量
} Stack;
// 初始化栈
void StackInit(Stack* ps) {
assert(ps);
STDataType* ptr = (STDataType*)(malloc(sizeof(STDataType) * STACK_SIZE));
if (ptr == NULL) {
printf("初始化失败!\n");
exit(-1);
}
ps->base = ptr;
ps->top = ptr;
ps->stackSize = STACK_SIZE;
}
// 扩容判断
void CheckCapacity(Stack* ps) {
assert(ps);
if (ps->top - ps->base >= ps->stackSize) {
int newSize = ps->stackSize * 2;
STDataType* newPtr = (STDataType*)(realloc(ps->base, sizeof(STDataType) * newSize));
if (newPtr == NULL) {
printf("扩容失败!\n");
exit(-1);
}
ps->base = newPtr;
ps->top = ps->base + ps->stackSize;
ps->stackSize = newSize;
}
}
// 入栈
void StackPush(Stack* ps, STDataType data) {
assert(ps);
CheckCapacity(ps);
*(ps->top) = data;
++(ps->top);
}
// 出栈
void StackPop(Stack* ps) {
assert(ps);
if (ps->top == ps->base) {
printf("栈为空,出栈失败!\n");
exit(-1);
}
--(ps->top);
}
// 获取栈顶元素
STDataType StackTop(Stack* ps) {
assert(ps);
if (ps->top == ps->base) {
printf("栈为空,获取栈顶元素失败!\n");
exit(-1);
}
return *(ps->top - 1);
}
// 获取栈中有效元素个数
int StackSize(Stack* ps) {
assert(ps);
return ps->top - ps->base;
}
// 检测栈是否为空,如果为空返回非零结果,如果不为空返回0
int StackEmpty(Stack* ps) {
assert(ps);
return ps->top == ps->base;
}
// 销毁栈
void StackDestroy(Stack* ps) {
assert(ps);
free(ps->base);
ps->base = NULL;
ps->top = NULL;
ps->stackSize = 0;
}
int main() {
Stack s;
StackInit(&s);
StackPush(&s, 1);
StackPush(&s, 2);
StackPush(&s, 3);
StackPush(&s, 4);
printf("栈顶元素为:%d\n", StackTop(&s)); // 输出:4
printf("栈中有效元素个数为:%d\n", StackSize(&s)); // 输出:4
StackPop(&s);
printf("栈顶元素为:%d\n", StackTop(&s)); // 输出:3
printf("栈中有效元素个数为:%d\n", StackSize(&s)); // 输出:3
StackDestroy(&s);
return 0;
}
```
阅读全文
相关推荐








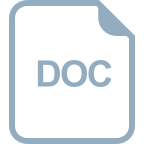





