统计不同字符数 【问题描述】 给定一个字符串,请你找出其中不含有重复字符的最长子串的长度。 Given a string, Please find out the length of the longest substring that does not contain repeated characters. 提示:相同字符大小写算做两个不同字符,如Aa为2 【输入形式】 字符串的内容 【输出形式】 不含有重复字符的最长子串的长度 【样例输入】 abcabcbb 【样例输出】 3
时间: 2023-10-14 07:11:23 浏览: 68
好的,这就是队列4-统计不同字符数的问题,非常感谢您的提醒和纠正。下面是 Python 的解法,使用双指针和哈希表来记录子串中出现的字符及其出现的次数:
```python
def length_of_longest_substring(s: str) -> int:
left, right = 0, 0
freq = {}
res = 0
while right < len(s):
c = s[right]
if c in freq:
freq[c] += 1
else:
freq[c] = 1
while freq[c] > 1:
d = s[left]
freq[d] -= 1
left += 1
res = max(res, right - left + 1)
right += 1
return res
```
您可以将输入字符串作为参数传递给 `length_of_longest_substring` 函数,然后它会返回不含有重复字符的最长子串的长度。对于输入样例 `"abcabcbb"`,函数的返回值应该为 `3`。
相关问题
队列4-统计不同字符数 【问题描述】 给定一个字符串,请你找出其中不含有重复字符的最长子串的长度。 Given a string, Please find out the length of the longest substring that does not contain repeated characters. 提示:相同字符大小写算做两个不同字符,如Aa为2 【输入形式】 字符串的内容 【输出形式】 不含有重复字符的最长子串的长度 【样例输入】 abcabcbb 【样例输出】 3
您好,这个问题和您给出的题目不太一样呢。根据您的要求,我可以提供一个 Python 的解法,如下所示:
```python
def length_of_longest_substring(s: str) -> int:
# 用一个字典记录每个字符上一次出现的位置
last_seen = {}
left = 0 # 不含重复字符的子串的左端点
res = 0 # 不含重复字符的子串的最大长度
for i, c in enumerate(s):
if c in last_seen and last_seen[c] >= left:
# 如果当前字符出现过,而且上一次出现的位置在当前子串中,更新左端点
left = last_seen[c] + 1
last_seen[c] = i # 记录当前字符出现的位置
res = max(res, i - left + 1) # 更新最大长度
return res
```
您可以将输入字符串作为参数传递给 `length_of_longest_substring` 函数,然后它会返回不含有重复字符的最长子串的长度。比如对于输入样例 `"abcabcbb"`,函数的返回值应该为 `3`。
用C语言队列实现:【问题描述】 给定一个字符串,请你找出其中不含有重复字符的最长子串的长度。 Given a string, Please find out the length of the longest substring that does not contain repeated characters. 提示:相同字符大小写算做两个不同字符,如Aa为2 【输入形式】 字符串的内容 【输出形式】 不含有重复字符的最长子串的长度 【样例输入】 abcabcbb 【样例输出】 3
以下是用C语言队列实现的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_QUEUE_SIZE 1000
typedef struct {
char data[MAX_QUEUE_SIZE];
int front;
int rear;
} Queue;
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
int isQueueEmpty(Queue *q) {
return q->front == q->rear;
}
int isQueueFull(Queue *q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
void enqueue(Queue *q, char c) {
if (isQueueFull(q)) {
fprintf(stderr, "Error: queue is full\n");
return;
}
q->data[q->rear] = c;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
char dequeue(Queue *q) {
if (isQueueEmpty(q)) {
fprintf(stderr, "Error: queue is empty\n");
return '\0';
}
char c = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return c;
}
int maxLengthSubstring(char *s) {
int n = strlen(s);
int maxLen = 0;
Queue q;
initQueue(&q);
for (int i = 0; i < n; i++) {
char c = s[i];
int j;
for (j = q.front; j != q.rear; j = (j + 1) % MAX_QUEUE_SIZE) {
if (q.data[j] == c) {
break;
}
}
if (j != q.rear) {
// found repeated character, dequeue until no repeat
while (!isQueueEmpty(&q) && q.data[q.front] != c) {
dequeue(&q);
}
dequeue(&q);
}
enqueue(&q, c);
int len = q.rear - q.front;
if (len > maxLen) {
maxLen = len;
}
}
return maxLen;
}
int main() {
char s[1000];
scanf("%s", s);
printf("%d\n", maxLengthSubstring(s));
return 0;
}
```
算法思路:
使用队列维护一个滑动窗口,窗口内的字符都是不重复的。遍历字符串,对于每个字符,判断是否在窗口内出现过,如果是则将队列中该字符之前的所有字符出队,直到队列中不含有该字符,然后将该字符入队,更新最长不重复子串的长度。
阅读全文
相关推荐
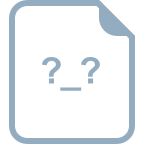
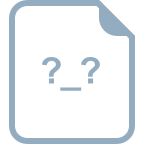
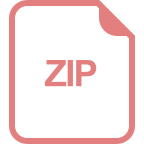








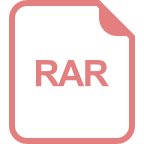
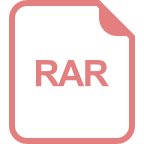
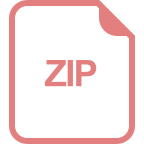