请设计easyx小游戏,使用c语言
时间: 2023-12-30 13:04:10 浏览: 138
好的,我可以为您设计一个简单的EasyX小游戏。这个游戏是一个打气球的小游戏,玩家需要点击屏幕上的气球,以获得分数。以下是游戏的详细设计:
1. 游戏开始后,程序会在屏幕上随机生成一些气球,气球的颜色、大小、位置等都是随机的。
2. 玩家需要用鼠标点击气球,点击到气球后,气球会爆炸并消失,同时玩家得到一定的分数。
3. 游戏的时间限制为60秒,60秒后游戏结束,并显示玩家的得分。
4. 如果玩家在60秒内没有点击到任何气球,游戏也会结束,并显示玩家的得分。
5. 在游戏结束后,玩家可以选择重新开始游戏或退出游戏。
以下是游戏的代码实现:
```c
#include <graphics.h>
#include <conio.h>
#include <time.h>
int main()
{
// 初始化随机数种子
srand((unsigned)time(NULL));
// 初始化图形界面
initgraph(640, 480);
// 设置字体
settextstyle(20, 0, _T("宋体"));
// 定义变量
int score = 0; // 得分
int timeLeft = 60; // 剩余时间
// 游戏循环
while (timeLeft > 0)
{
// 生成气球
int x = rand() % 560 + 40; // x坐标
int y = rand() % 360 + 40; // y坐标
int r = rand() % 30 + 10; // 半径
COLORREF color = RGB(rand() % 256, rand() % 256, rand() % 256); // 颜色
// 绘制气球
setfillcolor(color);
setlinecolor(BLACK);
fillcircle(x, y, r);
circle(x, y, r);
// 等待玩家点击
while (!MouseHit())
{
if (timeLeft > 0)
{
// 显示倒计时
TCHAR strTime[10];
_stprintf_s(strTime, _T("Time: %d"), timeLeft);
outtextxy(10, 10, strTime);
// 显示得分
TCHAR strScore[10];
_stprintf_s(strScore, _T("Score: %d"), score);
outtextxy(10, 40, strScore);
// 延时1秒
Sleep(1000);
// 更新时间
timeLeft--;
}
else
{
break;
}
}
// 处理玩家点击
if (MouseHit())
{
MOUSEMSG msg = GetMouseMsg();
if (msg.uMsg == WM_LBUTTONDOWN)
{
// 判断是否击中气球
if (sqrt(pow(msg.x - x, 2) + pow(msg.y - y, 2)) <= r)
{
// 加分
score += 10;
// 爆炸效果
setlinecolor(color);
circle(x, y, r * 1.2);
Sleep(50);
setlinecolor(BLACK);
circle(x, y, r * 1.2);
}
}
}
// 清空鼠标消息
FlushMouseMsgBuffer();
// 清空屏幕
cleardevice();
}
// 显示游戏结束画面
cleardevice();
settextstyle(40, 0, _T("宋体"));
outtextxy(200, 200, _T("Game Over"));
TCHAR strScore[20];
_stprintf_s(strScore, _T("Your Score: %d"), score);
outtextxy(200, 250, strScore);
settextstyle(20, 0, _T("宋体"));
outtextxy(200, 300, _T("Press Enter to Restart, Esc to Exit"));
// 等待玩家选择
while (1)
{
if (_kbhit())
{
int key = _getch();
if (key == VK_RETURN)
{
// 重新开始游戏
score = 0;
timeLeft = 60;
break;
}
else if (key == VK_ESCAPE)
{
// 退出游戏
closegraph();
return 0;
}
}
}
// 关闭图形界面
closegraph();
return 0;
}
```
这个游戏并不是很复杂,但是足以展示EasyX和C语言开发小游戏的基本流程。希望对您有所帮助!
阅读全文
相关推荐
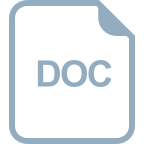
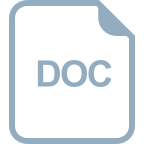
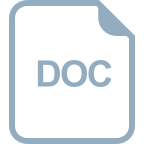
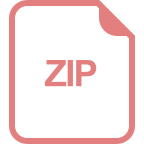
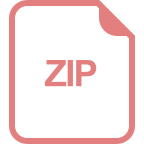
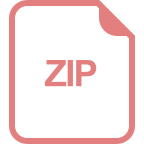
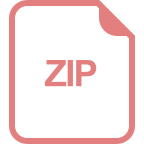
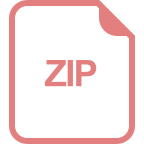
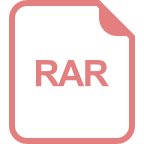
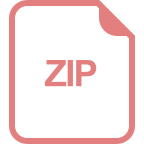
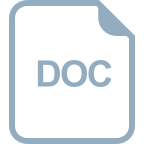
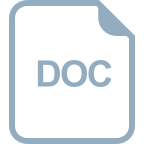





