请在这个DeepCFD的网络添加attention机制,并给出示例代码:import paddle import paddle.nn as nn class Attention(nn.Layer): def __init__(self, input_size, hidden_size): super(Attention, self).__init__() self.input_size = input_size self.hidden_size = hidden_size self.attn = nn.Linear(self.input_size + self.hidden_size, 1) self.softmax = nn.Softmax(axis=1) def forward(self, input, hidden): max_len = input.shape[0] attn_energies = paddle.zeros([max_len, 1]) for i in range(max_len): attn_energies[i] = self.score(input[i], hidden) attn_weights = self.softmax(attn_energies) context = paddle.sum(attn_weights * input, axis=0) return context.unsqueeze(0) def score(self, input, hidden): energy = self.attn(paddle.concat([input, hidden], axis=1)) return energy class DeepCFD(nn.Layer): def __init__(self, input_size, hidden_size, output_size): super(DeepCFD, self).__init__() self.input_size = input_size self.hidden_size = hidden_size self.output_size = output_size self.lstm = nn.LSTM(input_size, hidden_size, num_layers=2, batch_first=True) self.attention = Attention(input_size, hidden_size) self.fc = nn.Linear(hidden_size, output_size) def forward(self, input): output, (hidden, cell) = self.lstm(input) context = self.attention(output, hidden[-1]) output = self.fc(context) return output
时间: 2023-06-16 07:03:09 浏览: 230
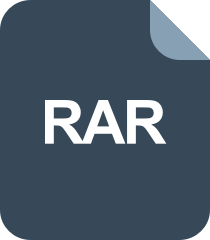
添加attention机制的LSTM时间序列预测(matlab)

好的,下面是添加了Attention机制的DeepCFD网络的示例代码:
import paddle
import paddle.nn as nn
class Attention(nn.Layer):
def __init__(self, input_size, hidden_size):
super(Attention, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.attn = nn.Linear(self.input_size + self.hidden_size, 1)
self.softmax = nn.Softmax(axis=1)
def forward(self, input, hidden):
max_len = input.shape[1]
attn_energies = paddle.zeros([input.shape[0], max_len, 1])
for i in range(max_len):
attn_energies[:, i] = self.score(input[:, i, :], hidden)
attn_weights = self.softmax(attn_energies)
context = paddle.sum(attn_weights * input, axis=1)
return context
def score(self, input, hidden):
energy = self.attn(paddle.concat([input, hidden], axis=1))
return energy
class DeepCFD(nn.Layer):
def __init__(self, input_size, hidden_size, output_size):
super(DeepCFD, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.lstm = nn.LSTM(input_size, hidden_size, num_layers=2, batch_first=True)
self.attention = Attention(input_size, hidden_size)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, input):
output, (hidden, cell) = self.lstm(input)
context = self.attention(output, hidden[-1])
output = self.fc(context)
return output
在这个示例代码中,我们将Attention机制应用到了LSTM的输出上。在Attention中,我们计算了每个时间步的注意力能量,然后使用softmax函数计算注意力权重。然后,我们将这些权重与LSTM输出相乘并求和,得到上下文向量作为Attention机制的输出。
在DeepCFD中,我们使用了两层LSTM,然后将LSTM输出和最后一个时刻的隐藏状态作为Attention机制的输入。最后,我们将Attention机制的输出传递到一个全连接层中,得到最终的输出。
阅读全文
相关推荐
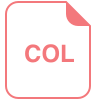
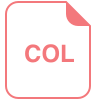
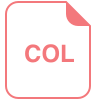
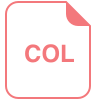





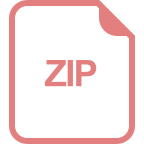
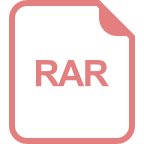
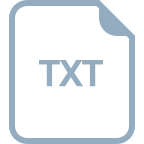
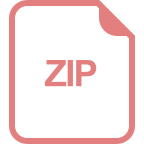
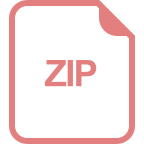
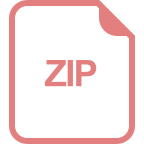