非递归方法实现汉诺塔问题的C语言代码
时间: 2024-05-07 18:16:55 浏览: 8
下面是非递归方式实现汉诺塔问题的C语言代码:
```C
#include <stdio.h>
#include <stdlib.h>
#define MaxSize 1000
#define ElemType int
//定义栈结构
typedef struct {
int top;
ElemType A[MaxSize];
} Stack;
//初始化栈
void InitStack(Stack *S) {
S->top = 0;
}
//判断栈是否为空
int IsEmptyStack(Stack *S) {
return S->top == 0;
}
//判断栈是否已满
int IsFullStack(Stack *S) {
return S->top == MaxSize;
}
//入栈
void Push(Stack *S, ElemType e) {
if (IsFullStack(S)) {
printf("Stack is full!\n");
exit(1);
} else {
S->A[S->top++] = e;
}
}
//出栈
ElemType Pop(Stack *S) {
if (IsEmptyStack(S)) {
printf("Stack is empty!\n");
exit(1);
} else {
return S->A[--S->top];
}
}
//非递归方式实现汉诺塔
void Hanoi(int n) {
Stack *S = (Stack *) malloc(sizeof(Stack));
InitStack(S);
int i;
for (i = n; i >= 1; i--) {
Push(S, i);
}
int j;
ElemType x, y;
for (j = 1; j <= (1 << n) - 1; j++) {
if (j % 3 == 1) {
x = Pop(S);
printf("%d: Move disk %d from A to C\n", j, x);
Push(S, x);
} else if (j % 3 == 2) {
y = Pop(S);
printf("%d: Move disk %d from A to B\n", j, y);
x = Pop(S);
printf("%d: Move disk %d from A to C\n", j, x);
Push(S, y);
Push(S, x);
} else {
y = Pop(S);
printf("%d: Move disk %d from B to C\n", j, y);
Push(S, y);
}
}
free(S);
}
//主函数
int main() {
int n = 3;
Hanoi(n);
return 0;
}
```
此代码使用栈实现了汉诺塔问题的非递归解法。每次移动都是在栈中进行的,而不是通过递归调用函数实现。当然,这只是一种特殊的解法,使用递归调用函数依然是汉诺塔问题的标准解法。
相关推荐
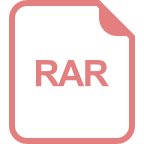














