一个由tkinter按钮指令控制的封装成函数的pygame俄罗斯方块小游戏
时间: 2023-06-20 18:06:41 浏览: 46
以下是一个封装成函数的pygame俄罗斯方块小游戏示例代码。该代码使用tkinter按钮指令控制游戏的开始和暂停。
```python
import pygame
import tkinter as tk
# 游戏区域大小
WIDTH = 300
HEIGHT = 600
# 方块大小
BLOCK_SIZE = 30
# 游戏区域左上角坐标
GAME_X = 20
GAME_Y = 20
# 游戏区域行列数
ROWS = HEIGHT // BLOCK_SIZE
COLS = WIDTH // BLOCK_SIZE
# 定义方块类
class Block(pygame.sprite.Sprite):
def __init__(self, color):
super().__init__()
self.color = color
self.image = pygame.Surface((BLOCK_SIZE, BLOCK_SIZE))
self.image.fill(self.color)
self.rect = self.image.get_rect()
def update(self, x, y):
self.rect.x = x
self.rect.y = y
# 定义俄罗斯方块类
class Tetromino(pygame.sprite.Group):
def __init__(self, color, x, y):
super().__init__()
self.color = color
self.x = x
self.y = y
def create_tetromino(self, shape):
self.shape = shape
self.blocks = []
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 'x':
block = Block(self.color)
block.update(self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE)
self.blocks.append(block)
self.add(block)
def move_down(self):
self.y += BLOCK_SIZE
for block in self.blocks:
block.update(block.rect.x, block.rect.y + BLOCK_SIZE)
def move_left(self):
self.x -= BLOCK_SIZE
for block in self.blocks:
block.update(block.rect.x - BLOCK_SIZE, block.rect.y)
def move_right(self):
self.x += BLOCK_SIZE
for block in self.blocks:
block.update(block.rect.x + BLOCK_SIZE, block.rect.y)
def rotate(self):
self.blocks = []
self.remove(self)
self.shape = list(zip(*self.shape[::-1]))
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 'x':
block = Block(self.color)
block.update(self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE)
self.blocks.append(block)
self.add(block)
# 定义游戏类
class Game:
def __init__(self):
# 初始化pygame和游戏区域
pygame.init()
pygame.display.set_caption("Tetris")
self.screen = pygame.display.set_mode((WIDTH + 2 * GAME_X, HEIGHT + 2 * GAME_Y))
self.clock = pygame.time.Clock()
self.font = pygame.font.Font(None, 30)
self.game_over = False
# 初始化游戏区域矩阵
self.grid = [[0 for _ in range(COLS)] for _ in range(ROWS)]
# 初始化方块组和当前俄罗斯方块
self.all_blocks = pygame.sprite.Group()
self.current_tetromino = None
# 游戏循环
def run(self):
while not self.game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.current_tetromino.move_left()
elif event.key == pygame.K_RIGHT:
self.current_tetromino.move_right()
elif event.key == pygame.K_UP:
self.current_tetromino.rotate()
elif event.key == pygame.K_DOWN:
self.current_tetromino.move_down()
# 更新当前俄罗斯方块位置
self.current_tetromino.move_down()
# 检测碰撞
if self.check_collision():
# 将当前俄罗斯方块加入游戏区域矩阵和方块组,创建新的俄罗斯方块
self.update_grid()
self.create_new_tetromino()
# 绘制游戏区域
self.draw()
# 更新屏幕
pygame.display.update()
# 控制游戏帧率
self.clock.tick(30)
# 开始游戏
def start(self):
self.create_new_tetromino()
self.run()
# 暂停游戏
def pause(self):
self.game_over = True
# 检测碰撞
def check_collision(self):
for block in self.current_tetromino.blocks:
row = (block.rect.y - GAME_Y) // BLOCK_SIZE
col = (block.rect.x - GAME_X) // BLOCK_SIZE
if row >= ROWS or col < 0 or col >= COLS or self.grid[row][col]:
return True
return False
# 将当前俄罗斯方块加入游戏区域矩阵和方块组,创建新的俄罗斯方块
def update_grid(self):
for block in self.current_tetromino.blocks:
row = (block.rect.y - GAME_Y) // BLOCK_SIZE
col = (block.rect.x - GAME_X) // BLOCK_SIZE
self.grid[row][col] = block
self.all_blocks.add(block)
self.current_tetromino = None
# 消除行
for i in range(ROWS - 1, -1, -1):
if all(self.grid[i]):
for block in self.grid[i]:
self.all_blocks.remove(block)
self.grid.pop(i)
self.grid.insert(0, [0 for _ in range(COLS)])
for row in range(1, i + 1):
for col in range(COLS):
if self.grid[row][col]:
block = self.grid[row][col]
self.grid[row - 1][col] = block
self.grid[row][col] = 0
block.update(block.rect.x, block.rect.y + BLOCK_SIZE)
# 创建新的俄罗斯方块
def create_new_tetromino(self):
tetrominoes = [
('I', (0, 255, 255), [[' ', ' ', ' ', ' '],
['x', 'x', 'x', 'x'],
[' ', ' ', ' ', ' '],
[' ', ' ', ' ', ' ']]),
('J', (0, 0, 255), [[' ', ' ', ' '],
['x', 'x', 'x'],
[' ', ' ', 'x']]),
('L', (255, 165, 0), [[' ', ' ', ' '],
['x', 'x', 'x'],
['x', ' ', ' ']]),
('O', (255, 255, 0), [['x', 'x'],
['x', 'x']]),
('S', (0, 255, 0), [[' ', ' ', ' '],
[' ', 'x', 'x'],
['x', 'x', ' ']]),
('T', (128, 0, 128), [[' ', ' ', ' '],
['x', 'x', 'x'],
[' ', 'x', ' ']]),
('Z', (255, 0, 0), [[' ', ' ', ' '],
['x', 'x', ' '],
[' ', 'x', 'x']]),
]
shape = tetrominoes[pygame.time.get_ticks() // 500 % len(tetrominoes)][2]
self.current_tetromino = Tetromino(tetrominoes[0][1], WIDTH // 2 - 2 * BLOCK_SIZE, GAME_Y)
self.current_tetromino.create_tetromino(shape)
if self.check_collision():
self.game_over = True
# 绘制游戏区域
def draw(self):
self.screen.fill((255, 255, 255))
pygame.draw.rect(self.screen, (0, 0, 0), (GAME_X - 1, GAME_Y - 1, WIDTH + 2, HEIGHT + 2), 1)
for row in range(ROWS):
for col in range(COLS):
block = self.grid[row][col]
if block:
pygame.draw.rect(self.screen, block.color, (block.rect.x, block.rect.y, BLOCK_SIZE, BLOCK_SIZE))
self.all_blocks.draw(self.screen)
if self.game_over:
text = self.font.render("Game Over", True, (255, 0, 0))
self.screen.blit(text, ((WIDTH - text.get_width()) // 2, (HEIGHT - text.get_height()) // 2))
```
使用tkinter创建按钮控制游戏的开始和暂停:
```python
def start_game():
game = Game()
game.start()
def pause_game():
game.pause()
root = tk.Tk()
start_button = tk.Button(root, text="Start", command=start_game)
pause_button = tk.Button(root, text="Pause", command=pause_game)
start_button.pack()
pause_button.pack()
root.mainloop()
```
相关推荐
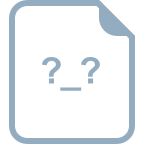
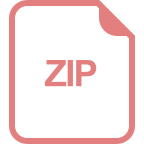
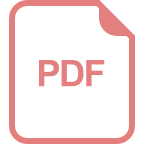



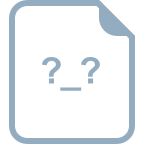
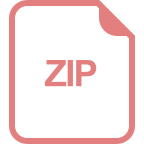
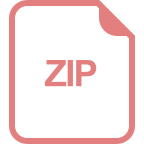
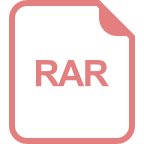
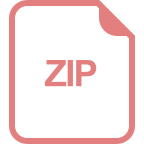
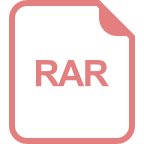
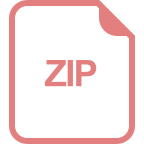