一个由tkinter按钮指令控制的封装成函数的pygame计分俄罗斯方块(方块可转动)小游戏
时间: 2023-06-19 09:09:21 浏览: 50
下面是一个由tkinter按钮指令控制的封装成函数的pygame计分俄罗斯方块小游戏的示例代码:
```python
import pygame, random
from pygame.locals import *
from tkinter import *
# 设置窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 500
# 方块大小
BLOCK_SIZE = 20
# 方块颜色
COLORS = [(255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (128, 0, 128)]
# 定义方块形状
SHAPES = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6, 6, 6]],
[[7, 7],
[7, 7]]
]
# 初始化pygame
pygame.init()
# 设置窗口标题
pygame.display.set_caption('俄罗斯方块')
# 创建窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 创建时钟
clock = pygame.time.Clock()
# 创建字体
font = pygame.font.SysFont(None, 36)
# 定义游戏状态
GAME_STATE_READY = 0
GAME_STATE_PLAYING = 1
GAME_STATE_GAME_OVER = 2
# 定义方块类
class Block:
def __init__(self, shape, color):
self.shape = shape
self.color = color
self.x = int(WINDOW_WIDTH / BLOCK_SIZE / 2 - len(shape[0]) / 2)
self.y = -len(shape)
# 绘制方块
def draw(self, surface):
for y in range(len(self.shape)):
for x in range(len(self.shape[0])):
if self.shape[y][x] != 0:
rect = pygame.Rect((self.x + x) * BLOCK_SIZE, (self.y + y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(surface, self.color, rect)
# 检查能否移动
def check_collision(self, board, dx, dy):
for y in range(len(self.shape)):
for x in range(len(self.shape[0])):
if self.shape[y][x] != 0:
if y + self.y + dy >= len(board) or x + self.x + dx < 0 or x + self.x + dx >= len(board[y]) or board[y + self.y + dy][x + self.x + dx] != 0:
return True
return False
# 移动方块
def move(self, board, dx, dy):
if not self.check_collision(board, dx, dy):
self.x += dx
self.y += dy
return True
return False
# 旋转方块
def rotate(self, board):
new_shape = []
for x in range(len(self.shape[0])):
new_row = []
for y in range(len(self.shape) - 1, -1, -1):
new_row.append(self.shape[y][x])
new_shape.append(new_row)
if not self.check_collision(board, 0, 0, new_shape):
self.shape = new_shape
# 创建游戏板
board = [[0] * int(WINDOW_WIDTH / BLOCK_SIZE) for i in range(int(WINDOW_HEIGHT / BLOCK_SIZE))]
# 创建方块
block = Block(random.choice(SHAPES), random.choice(COLORS))
# 初始化分数
score = 0
# 初始化游戏状态
game_state = GAME_STATE_READY
# 定义开始游戏函数
def start_game():
global game_state, score, board, block
game_state = GAME_STATE_PLAYING
score = 0
board = [[0] * int(WINDOW_WIDTH / BLOCK_SIZE) for i in range(int(WINDOW_HEIGHT / BLOCK_SIZE))]
block = Block(random.choice(SHAPES), random.choice(COLORS))
# 定义绘制游戏界面函数
def draw_game():
# 绘制游戏板
for y in range(len(board)):
for x in range(len(board[y])):
if board[y][x] != 0:
rect = pygame.Rect(x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(screen, COLORS[board[y][x] - 1], rect)
# 绘制方块
block.draw(screen)
# 绘制分数
score_text = font.render('Score: {}'.format(score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
# 定义游戏结束函数
def game_over():
global game_state
game_state = GAME_STATE_GAME_OVER
# 创建开始按钮
start_button = Button(text='开始游戏', command=start_game)
start_button.pack()
# 开始游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == KEYDOWN:
if game_state == GAME_STATE_PLAYING:
if event.key == K_LEFT:
block.move(board, -1, 0)
elif event.key == K_RIGHT:
block.move(board, 1, 0)
elif event.key == K_DOWN:
block.move(board, 0, 1)
elif event.key == K_UP:
block.rotate(board)
elif game_state == GAME_STATE_GAME_OVER:
if event.key == K_RETURN:
start_game()
# 绘制背景
screen.fill((0, 0, 0))
# 更新方块位置
if game_state == GAME_STATE_PLAYING:
if not block.move(board, 0, 1):
for y in range(len(block.shape)):
for x in range(len(block.shape[0])):
if block.shape[y][x] != 0:
board[block.y + y][block.x + x] = block.color[0] // 50 + 1
for y in range(len(board)):
if all(board[y]):
del board[y]
board.insert(0, [0] * len(board[0]))
score += 10
block = Block(random.choice(SHAPES), random.choice(COLORS))
if block.check_collision(board, 0, 0):
game_over()
# 绘制游戏界面
draw_game()
# 刷新画面
pygame.display.update()
# 控制帧率
clock.tick(30)
```
这个小游戏的主要思路是使用pygame实现俄罗斯方块的游戏逻辑,然后使用tkinter创建一个按钮,点击按钮后开始游戏。游戏界面会在pygame窗口中绘制,并且显示当前得分。按下方向键或向上键可以移动或旋转方块,当方块无法继续下落时,会被固定在游戏板上,然后判断是否有满行,如果有,则消除该行,得分增加。如果新生成的方块无法放置在游戏板上,则游戏结束。
相关推荐
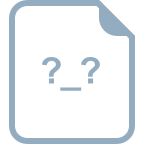
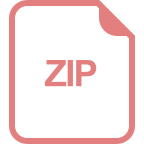
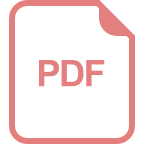










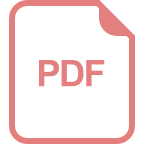
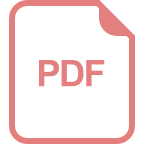
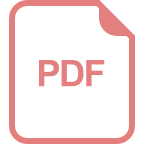
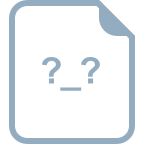