string path
时间: 2024-03-03 12:17:26 浏览: 19
在计算机编程中,`path`通常指的是文件或目录在文件系统中的路径。在Java语言中,`String path`通常是指文件或目录的路径名,可以用于创建和访问文件、目录,或者在文件系统中定位某个文件或目录。
在使用Java进行文件读写操作时,需要指定文件的路径。比如,如果要读取名为`example.txt`的文件,需要使用`String path = "example.txt"`来指定文件路径。如果文件位于程序所在目录下,则可以直接使用文件名来指定路径;如果文件在其他目录下,则需要使用绝对或相对路径来指定路径。
在使用Java中的一些API进行文件操作时,也需要使用`String path`来指定文件路径,比如`File`类的构造函数、`FileInputStream`和`FileOutputStream`等类的构造函数。
相关问题
String path : fileList
这段代码有误,应该是两个变量声明和初始化,而不是一个变量和一个数组的声明。应该改成以下形式:
```
String path = "fileList";
String[] fileList = {"file1.txt", "file2.txt", "file3.txt"};
```
其中,`path` 是字符串类型的变量,它的值是 `"fileList"`;`fileList` 是字符串数组类型的变量,它包含了三个元素 `"file1.txt"`、`"file2.txt"` 和 `"file3.txt"`。
string path = request.getconte
### 回答1:
xtPath();
这是一个Java代码片段,其中request是一个HttpServletRequest对象,getcontextPath()是该对象的一个方法,用于获取当前Web应用程序的上下文路径。该方法返回一个字符串,表示当前Web应用程序的上下文路径。例如,如果Web应用程序的上下文路径为“/myapp”,则该方法返回字符串“/myapp”。
### 回答2:
request.getconte是一个错误的方法或属性。在常见的编程语言中,例如Java或Python,request对象通常用于处理HTTP请求,并提供了一组方法和属性来访问请求的不同方面,例如请求头、请求体等。
通常,要获取请求路径,应使用request对象的get请求路径属性或方法,例如request.getPath()或request.path。
例如,如果使用Java语言,可以使用HttpServletRequest对象来获取请求路径。正确的代码如下:
```java
String path = request.getPath(); //获取请求的路径
```
如果使用Python语言和Flask框架,可以使用request对象的path属性来获取请求路径。正确的代码如下:
```python
path = request.path #获取请求的路径
```
总之,根据具体的编程语言和框架,需要使用正确的方法或属性来获取请求路径。在给定的代码中,request.getconte是错误的,应该使用正确的方法或属性来获取请求的路径。
相关推荐
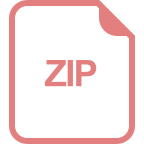
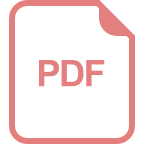
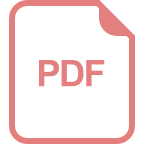













