list<T> contains
时间: 2023-08-15 22:07:32 浏览: 57
The `std::list<T>` container in C++ is a doubly-linked list implementation where each element is stored in a separate node. The `list` container offers constant time insertion and removal of elements from anywhere in the container, but accessing elements by index requires linear time.
To check if a `list` contains a specific element, you can use the `std::find` algorithm from the `<algorithm>` header. Here's an example:
```
#include <iostream>
#include <list>
#include <algorithm>
int main() {
std::list<int> mylist = {1, 2, 3, 4, 5};
int to_find = 3;
if (std::find(mylist.begin(), mylist.end(), to_find) != mylist.end()) {
std::cout << "Found " << to_find << " in the list!\n";
} else {
std::cout << to_find << " not found in the list.\n";
}
return 0;
}
```
In this example, we create a `list` of integers `mylist` and initialize it with values 1 through 5. We want to check if the `list` contains the value 3, so we use `std::find` to search for it. If `std::find` returns an iterator pointing to the end of the `list`, it means the element was not found. Otherwise, we output a message saying we found the element.
相关推荐
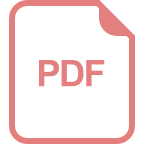













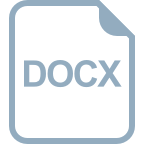