torch.no_grad
时间: 2023-08-07 11:41:02 浏览: 43
`torch.no_grad()` 是一个上下文管理器,用于在不需要计算梯度时禁用梯度计算,以提高代码效率。在使用 `torch.autograd` 计算梯度时,每个操作都会产生梯度,如果在不需要计算梯度的情况下进行操作,会浪费计算资源,同时也可能会导致出错。
使用 `torch.no_grad()` 可以在临时禁用梯度计算的情况下进行操作,从而提高代码效率。例如,可以使用 `torch.no_grad()` 包装测试代码,以避免计算测试时的梯度,从而提高测试速度和准确性。
下面是一个使用 `torch.no_grad()` 的例子:
```python
import torch
x = torch.tensor([1.0], requires_grad=True)
y = x ** 2
with torch.no_grad():
z = y * 2
print(z) # tensor([2.])
grad_y = torch.autograd.grad(y, x)[0] # 计算 y 对 x 的梯度
print(grad_y) # tensor([2.])
```
相关问题
torch.no_grad()
torch.no_grad() is a context manager that is used to turn off gradient calculation during the forward pass of a neural network. This is useful when you are only interested in using a trained model for inference or prediction, and do not need to calculate gradients or update the model's weights during this process. By disabling gradient calculation, you can save memory and computation time.
For example, consider the following code:
```
with torch.no_grad():
output = model(input)
```
In this code, we use the no_grad() context manager to disable gradient calculation for the forward pass of the model. This means that any operations that require gradients, such as backward() or optimizer.step(), will not be executed. This is useful when you want to use the model for inference, but not for training.
Note that using no_grad() does not affect the state of the model, so you can still call model.eval() to put the model in evaluation mode and use it for inference.
with torch.no_grad的作用
torch.no_grad() 是一个上下文管理器,用于在代码块中临时禁用梯度计算。当我们不需要计算梯度时,可以使用 torch.no_grad() 来提高代码的执行效率。
在深度学习中,梯度计算是反向传播算法的关键步骤。然而,在推理阶段或者对模型进行评估时,并不需要计算梯度,只需要使用模型的前向传播结果。此时,通过使用 torch.no_grad() 可以避免不必要的内存消耗和计算开销。
当进入 torch.no_grad() 的上下文环境后,所有位于该环境中的操作都不会被记录用于自动求导,也不会构建计算图。这样可以减少内存的消耗,加快代码的执行速度。
例如,在模型推理阶段,我们可以使用 torch.no_grad() 来包装前向传播的代码,以提高推理速度:
```python
with torch.no_grad():
output = model(input)
```
在上述代码中,模型的前向传播过程不会被记录用于自动求导,从而提高了推理阶段的效率。
相关推荐
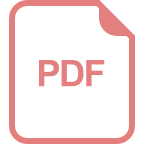
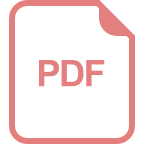








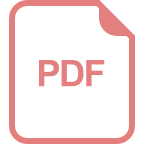
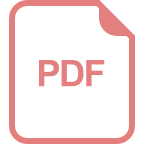
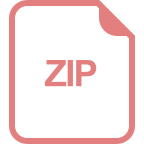