torch.no_grad():
时间: 2024-06-08 14:08:00 浏览: 20
torch.no_grad()是PyTorch中的一个上下文管理器,用于在代码块中关闭梯度跟踪。在使用torch.no_grad()包裹的代码块中,PyTorch将不会跟踪这些操作的梯度信息,从而减少了计算和内存消耗。
具体使用方法是在代码块外部使用`with torch.no_grad():`将需要关闭梯度跟踪的代码包裹起来。在这个代码块中,所有的操作都不会对梯度进行跟踪和计算。
举个例子,如果我们有一个张量x和一个张量w,我们想要计算它们的乘积y,并且不希望计算y的梯度,我们可以使用torch.no_grad()来实现:
```
import torch
x = torch.tensor([2.0], requires_grad=True)
w = torch.tensor([3.0], requires_grad=True)
with torch.no_grad():
y = x * w
```
在这个例子中,我们通过将x和w张量的`requires_grad`属性设置为True,表示我们希望计算它们的梯度。然而,在torch.no_grad()的上下文中,对于y的计算不会被跟踪,也不会生成梯度信息。因此,在执行`y.backward()`时会报错。
总结来说,torch.no_grad()是一种在特定代码块中关闭梯度跟踪的方法,可以在训练阶段使用,以减少计算和内存消耗。
相关问题
torch.no_grad
`torch.no_grad()` 是一个上下文管理器,用于在不需要计算梯度时禁用梯度计算,以提高代码效率。在使用 `torch.autograd` 计算梯度时,每个操作都会产生梯度,如果在不需要计算梯度的情况下进行操作,会浪费计算资源,同时也可能会导致出错。
使用 `torch.no_grad()` 可以在临时禁用梯度计算的情况下进行操作,从而提高代码效率。例如,可以使用 `torch.no_grad()` 包装测试代码,以避免计算测试时的梯度,从而提高测试速度和准确性。
下面是一个使用 `torch.no_grad()` 的例子:
```python
import torch
x = torch.tensor([1.0], requires_grad=True)
y = x ** 2
with torch.no_grad():
z = y * 2
print(z) # tensor([2.])
grad_y = torch.autograd.grad(y, x)[0] # 计算 y 对 x 的梯度
print(grad_y) # tensor([2.])
```
torch的.no_grad
在PyTorch中,`torch.no_grad()`是一个上下文管理器,用于控制是否计算梯度。当我们在一个`with torch.no_grad():`块内时,PyTorch会停止自动计算梯度,这对于我们不需要梯度的推理过程非常有用。
在这个上下文管理器中的操作将不会产生梯度。这意味着,通过此块内的计算得到的张量将不具有`requires_grad`属性,也没有与之相关的梯度函数(`grad_fn`)。这可以帮助我们减少内存消耗并提高推理速度。
在深度学习中,我们通常使用`torch.no_grad()`块执行模型评估或推理,因为我们不需要计算梯度,而只是对模型进行前向传播。
总结来说,`torch.no_grad()`是一个PyTorch上下文管理器,用于停止自动计算梯度。在此上下文管理器中的计算不会产生梯度,并且相关的张量也不具有`requires_grad`属性和梯度函数。
相关推荐
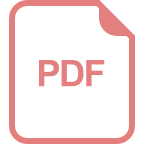
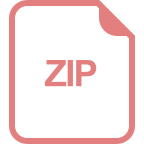
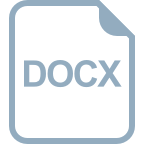




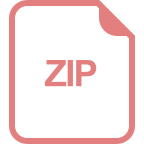
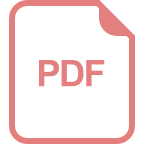
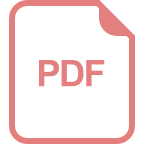
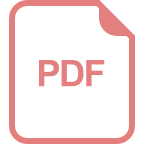
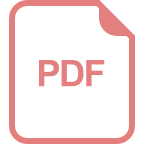
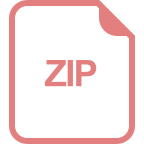
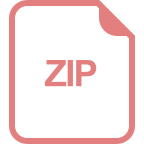