void fun(File source,File dest) { try(FileInputStream fis = new FileInputStream(source); FileOutputStream fos = new FileOutputStream(dest)){ byte[] bs = new byte[1024]; int n; while((n = fis.read(bs)) != -1) { fos.write(bs, 0, n); } } catch (IOException e) { e.printStackTrace(); } } 上面方法的功能是?
时间: 2024-04-21 12:30:04 浏览: 98
上面的方法功能是将源文件复制到目标文件中。具体实现是通过创建FileInputStream对象读取源文件,创建FileOutputStream对象写入目标文件,并使用缓存数组来提高复制效率。在复制过程中,程序每次最多读取1024字节,然后将其写入目标文件中,直到源文件读取完毕。如果在复制过程中发生了异常,则会输出异常信息。
相关问题
public boolean copyFile(String src, String dest) throws IOException { boolean flag = false; if (!flag) { File srcFile = new File(src); File destFile = new File(dest); FileInputStream fis = new FileInputStream(srcFile); FileOutputStream fos = new FileOutputStream(destFile); BufferedInputStream bis = new BufferedInputStream(fis); BufferedOutputStream bos = new BufferedOutputStream(fos, 1024); int len = 0; byte[] bytes = new byte[1024]; while ((len = bis.read(bytes)) != -1) bos.write(bytes, 0, len); bos.flush(); bis.close(); bos.close(); flag = true; } return flag; }
这是一个用于复制文件的Java方法,它会将指定的源文件src复制到目标文件dest。该方法使用了字节流的方式读写文件,并且使用了缓冲流来提高读写效率。在方法中,首先会检查flag变量的值,如果为false,表示可以执行文件复制操作,否则直接返回。然后创建源文件和目标文件的File对象,并使用FileInputStream和FileOutputStream来创建读写文件的输入输出流。接着,使用BufferedInputStream和BufferedOutputStream来创建缓冲流,将字节数据读入缓冲区,再从缓冲区写入到目标文件中。最后,关闭输入输出流和缓冲流,将flag变量设置为true,并返回该变量。
1、 编写一个程序,分别使用字节流和字符流拷贝一个文本文件。 提示: 需要在当前路径下,先建立一个src.txt的文本文件,内容如下图所示,然后通过文件字节输出流FileOutputStream在当前路径下建立dest1.txt文本文件,并且写入数据。通过文件字符输出流FileWriter在当前路径下建立dest2.txt文本文件,并且写入数据。 1) 使用FileInputStream、FileOutputStream和FileReader、FileWriter分别进行拷贝。 2) 使用字节流拷贝时,定义一个1024长度的字节数组作为缓冲区,使用字符流拷贝,使用BufferedReader和BufferedWriter包装流进行包装
好的,以下是使用字节流和字符流分别拷贝文本文件的代码示例:
1. 使用字节流拷贝文件
```java
import java.io.*;
public class ByteStreamCopy {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("src.txt");
FileOutputStream fos = new FileOutputStream("dest1.txt");
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fis.close();
fos.close();
System.out.println("拷贝完成!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
2. 使用字符流拷贝文件
```java
import java.io.*;
public class CharacterStreamCopy {
public static void main(String[] args) {
try {
FileReader fr = new FileReader("src.txt");
BufferedReader br = new BufferedReader(fr);
FileWriter fw = new FileWriter("dest2.txt");
BufferedWriter bw = new BufferedWriter(fw);
String line;
while ((line = br.readLine()) != null) {
bw.write(line);
bw.newLine();
}
br.close();
bw.close();
System.out.println("拷贝完成!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
注意:以上代码示例仅供参考,实际应用中需要对异常进行处理。
阅读全文
相关推荐
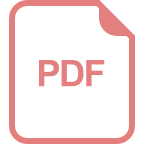
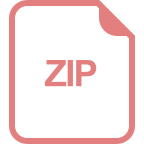
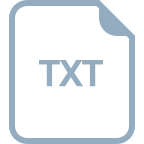
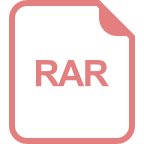
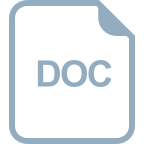
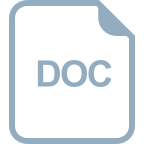
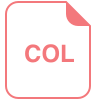










