编写一个程序,分别使用字节流和字符流拷贝一个文本文件。要求如下: (1)使用FileInputStream、FileOutputStreaem和FileReader、FileWriter分别进行拷贝。 (2)使用字节流拷贝时,定义一个1024长度的字节数组作为缓冲区;使用字符流拷贝,用java语言编写
时间: 2024-02-20 18:02:19 浏览: 119
好的,以下是使用字节流和字符流分别拷贝文件的示例代码:
使用字节流拷贝文件:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ByteStreamCopy {
public static void main(String[] args) {
String srcFile = "source.txt";
String destFile = "dest.txt";
byte[] buffer = new byte[1024];
int bytesRead;
try (FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile)) {
while ((bytesRead = fis.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
System.out.println("File copied successfully using byte stream");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
使用字符流拷贝文件:
```java
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class CharacterStreamCopy {
public static void main(String[] args) {
String srcFile = "source.txt";
String destFile = "dest.txt";
char[] buffer = new char[1024];
int charsRead;
try (FileReader fr = new FileReader(srcFile);
FileWriter fw = new FileWriter(destFile)) {
while ((charsRead = fr.read(buffer)) != -1) {
fw.write(buffer, 0, charsRead);
}
System.out.println("File copied successfully using character stream");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
注意:以上代码中的文件名和路径需要根据实际情况修改。
阅读全文
相关推荐
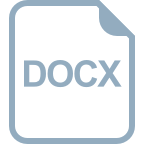













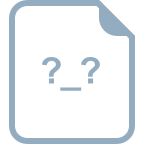