编写一个程序,分别使用字节流和字符流复制一个文件。要求如下: 1、使用FileInputStream、FileOutputStream和FileReader、FileWriter分别进行复制 2、使用字节流复制时,定义一个长度为1024的字节数组作为缓冲区。
时间: 2024-03-08 14:49:20 浏览: 162
好的,下面是使用字节流和字符流分别复制文件的示例代码:
使用字节流复制文件:
```java
import java.io.*;
public class ByteStreamCopy {
public static void main(String[] args) {
String srcFile = "source.txt";
String destFile = "destination.txt";
try (FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
System.out.println("File copied successfully using byte stream.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
使用字符流复制文件:
```java
import java.io.*;
public class CharacterStreamCopy {
public static void main(String[] args) {
String srcFile = "source.txt";
String destFile = "destination.txt";
try (FileReader reader = new FileReader(srcFile);
FileWriter writer = new FileWriter(destFile)) {
char[] buffer = new char[1024];
int length;
while ((length = reader.read(buffer)) > 0) {
writer.write(buffer, 0, length);
}
System.out.println("File copied successfully using character stream.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上两个示例代码中,都使用了try-with-resources语句来自动关闭文件流。在使用字节流复制文件时,定义了一个长度为1024的字节数组作为缓冲区,每次读取文件时将数据读入缓冲区,然后再将缓冲区中的数据写入目标文件。在使用字符流复制文件时,也定义了一个长度为1024的字符数组作为缓冲区,使用reader.read(buffer)方法读取文件数据,然后使用writer.write(buffer)方法将数据写入目标文件。
阅读全文
相关推荐
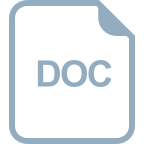
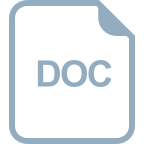
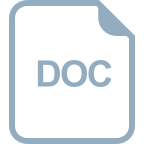












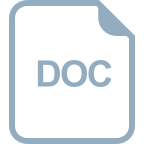
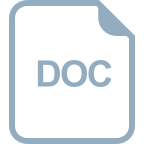