学习Java中的异常处理及常见的JavaAPI,然后编写一个程序,分别使用字节流和字符流拷贝一个文本文件。要求如下:(1)使用FileInputStream、FileOutputStreaem和Fil
时间: 2023-12-10 17:42:00 浏览: 38
eReader、FileWriter实现文件拷贝。(2)在文件拷贝时加入异常处理机制,保证程序的健壮性。以下是示例代码:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
String sourceFilePath = "source.txt";
String targetFilePath = "target.txt";
// 使用字节流拷贝文件
try (
FileInputStream fis = new FileInputStream(sourceFilePath);
FileOutputStream fos = new FileOutputStream(targetFilePath);
) {
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
System.out.println("文件拷贝完成!");
} catch (IOException e) {
System.out.println("文件拷贝失败: " + e.getMessage());
}
// 使用字符流拷贝文件
try (
FileReader fr = new FileReader(sourceFilePath);
FileWriter fw = new FileWriter(targetFilePath);
) {
char[] buffer = new char[1024];
int len;
while ((len = fr.read(buffer)) != -1) {
fw.write(buffer, 0, len);
}
System.out.println("文件拷贝完成!");
} catch (IOException e) {
System.out.println("文件拷贝失败: " + e.getMessage());
}
}
}
```
在上面的代码中,我们使用了 try-with-resources 语句来自动关闭输入输出流,避免了手动关闭流时可能出现的异常。同时,在文件拷贝时加入了异常处理机制,保证程序的健壮性。
相关推荐
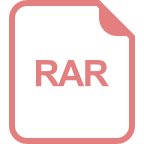
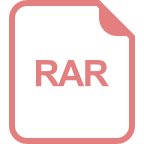
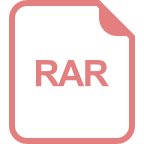
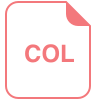
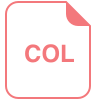
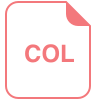
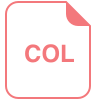
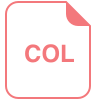










