用Java语言编写一个程序,分别使用字节流和字符流拷贝一个文本文件。要求如下:(1)使用 FileInputStream、FileOutputStreaem 和 FileReader、FileWriter 分别进行拷贝。 (2)使用字节流拷贝时,定义一个1024长度的字节数组作为缓冲区,使用字符流拷贝。
时间: 2024-03-25 10:36:43 浏览: 74
好的,以下是使用Java语言编写的程序,分别使用字节流和字符流拷贝一个文本文件:
```
import java.io.*;
public class TextFileCopy {
public static void main(String[] args) {
String sourceFilePath = "source.txt";
String byteCopyFilePath = "byteCopy.txt";
String charCopyFilePath = "charCopy.txt";
try {
// 使用 FileInputStream 和 FileOutputStream 进行字节流拷贝
FileInputStream fis = new FileInputStream(sourceFilePath);
FileOutputStream fos = new FileOutputStream(byteCopyFilePath);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fis.close();
fos.close();
System.out.println("Byte stream copy completed.");
// 使用 FileReader 和 FileWriter 进行字符流拷贝
FileReader fr = new FileReader(sourceFilePath);
FileWriter fw = new FileWriter(charCopyFilePath);
int c;
while ((c = fr.read()) != -1) {
fw.write(c);
}
fr.close();
fw.close();
System.out.println("Character stream copy completed.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的程序中,我们首先定义了三个文件路径变量,分别用于存储源文件、字节流拷贝文件和字符流拷贝文件的路径。
然后,我们使用 try-catch 块,分别实例化 FileInputStream、FileOutputStream、FileReader 和 FileWriter 对象。在字节流拷贝中,我们定义了一个长度为 1024 的字节数组作为缓冲区,使用 while 循环读取源文件内容,并将内容写入到字节流拷贝文件中。在字符流拷贝中,我们使用 while 循环读取源文件内容,并将内容写入到字符流拷贝文件中。
最后,我们关闭了所有的输入输出流,并输出了拷贝完成的信息。
希望这个程序能够帮到你!
阅读全文
相关推荐
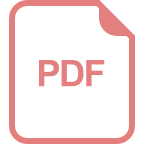











