求水洼数以及每个水洼的积水面积C语言代码
时间: 2024-11-20 15:39:15 浏览: 10
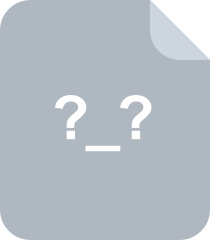
1.水洼数.c
在C语言中,求解水洼数和每个水洼的积水面积通常涉及到二维数组的问题,比如在一个地图上找出所有的小坑洼。这里我们可以使用深度优先搜索(DFS)算法来解决。以下是一个简单的示例代码:
```c
#include <stdio.h>
#define MAX_SIZE 100 // 地图大小
int map[MAX_SIZE][MAX_SIZE]; // 假设地图是一个整型二维数组
// 函数声明
void dfs(int row, int col, int visited[][MAX_SIZE], int &count, float &total_area);
int main() {
int size;
printf("请输入地图的行数:");
scanf("%d", &size);
// 初始化地图(假设所有元素为0,代表没有水)
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
map[i][j] = 0;
}
}
// 输入地图的具体情况(若有值非0,则表示有水洼)
printf("\n输入地图信息(非零表示有水洼):\n");
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
scanf("%d", &map[i][j]);
}
}
int count = 0; // 计数器,记录水洼数量
float total_area = 0.0f; // 总积水面积
int visited[MAX_SIZE][MAX_SIZE] = {0}; // 访问标记矩阵
// 遍历并应用DFS
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
if (!visited[i][j] && map[i][j] != 0) {
dfs(i, j, visited, count, total_area);
}
}
}
printf("\n水洼总数:%d\n", count);
printf("每个水洼的积水面积:%.2f\n", total_area / count);
return 0;
}
// DFS函数
void dfs(int row, int col, int visited[][MAX_SIZE], int &count, float &total_area) {
visited[row][col] = 1; // 标记当前位置已访问
// 检查周围的点
if (row > 0 && map[row - 1][col] == 0 && !visited[row - 1][col]) {
dfs(row - 1, col, visited, count, total_area);
}
if (row < size - 1 && map[row + 1][col] == 0 && !visited[row + 1][col]) {
dfs(row + 1, col, visited, count, total_area);
}
if (col > 0 && map[row][col - 1] == 0 && !visited[row][col - 1]) {
dfs(row, col - 1, visited, count, total_area);
}
if (col < size - 1 && map[row][col + 1] == 0 && !visited[row][col + 1]) {
dfs(row, col + 1, visited, count, total_area);
}
count++; // 当前访问到一个新的水洼,计数器加一
total_area += map[row][col]; // 积水面积累加
}
```
阅读全文
相关推荐
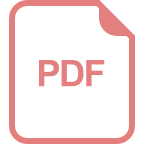
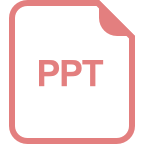







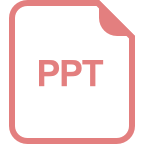
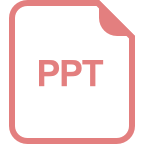
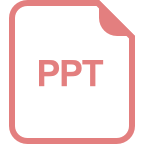
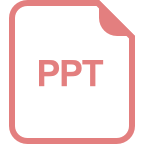