python zmq REQ和REP的几种用法
时间: 2024-06-01 11:14:26 浏览: 9
Python zmq的REQ和REP有三种用法:
1. 单向通信模式:REQ将请求发送给REP,等待REP回应。这种模式适用于请求和回应之间没有交互的情况。
2. 请求-应答模式:REQ将请求发送给REP,REP接收到请求后,回复一个响应。这种模式适用于请求和回应之间需要交互的情况。
3. 多路复用模式:REQ可以向多个REP发送请求,REP可以接收多个REQ发送的请求并回复响应。这种模式适用于多个客户端同时请求同一个服务端的情况。
相关问题
python zmq REQ和REP的请求-应答模式的代码
Sure, here is an example of Python code for the REQ-REP pattern using ZeroMQ (zmq) library:
REQ (client) side:
```python
import zmq
context = zmq.Context()
socket = context.socket(zmq.REQ)
socket.connect("tcp://localhost:5555")
socket.send(b"Hello")
message = socket.recv()
print("Received reply:", message)
```
REP (server) side:
```python
import zmq
context = zmq.Context()
socket = context.socket(zmq.REP)
socket.bind("tcp://*:5555")
while True:
message = socket.recv()
print("Received request:", message)
socket.send(b"World")
```
In this example, the client sends a "Hello" message to the server using REQ socket, and waits for a reply. The server receives the message using the REP socket, prints it out, and sends a "World" message back to the client. The client then prints out the server's reply.
Hope this helps!
python zmq的REQ和REP的代码示例
以下是Python中使用ZMQ REQ和REP模式的示例代码:
REQ端代码:
```python
import zmq
context = zmq.Context()
socket = context.socket(zmq.REQ)
socket.connect("tcp://localhost:5555")
while True:
message = input("请输入要发送的消息:")
socket.send_string(message)
response = socket.recv()
print("接收到的响应为:{}".format(response.decode()))
```
REP端代码:
```python
import zmq
context = zmq.Context()
socket = context.socket(zmq.REP)
socket.bind("tcp://*:5555")
while True:
message = socket.recv()
print("接收到的消息为:{}".format(message.decode()))
response = input("请输入要回复的消息:")
socket.send_string(response)
```
在这个示例中,REQ端通过创建一个ZMQ的Context对象和一个REQ类型的socket对象来与REP端通信。它使用socket.connect()方法来连接到REP端,然后通过socket.send_string()方法发送消息,使用socket.recv()方法接收响应。
REP端通过创建一个ZMQ的Context对象和一个REP类型的socket对象来接收REQ端的消息。它使用socket.bind()方法将socket对象绑定到一个特定的IP地址和端口号,然后使用socket.recv()方法接收REQ端发送的消息,并使用input()方法来回复消息,最后使用socket.send_string()方法将回复发送回REQ端。
相关推荐
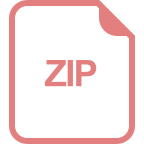
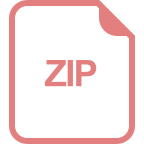
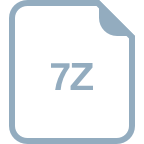












