请给出C语言 IPC消息队列 收发例子,并给出主要函数原型和参数说明
时间: 2024-02-03 17:15:36 浏览: 62
下面是一个简单的C语言IPC消息队列收发例子:
发送消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAXSIZE 128
struct msgbuf
{
long mtype;
char mtext[MAXSIZE];
};
int main()
{
key_t key;
int msgid;
struct msgbuf msg;
key = ftok(".", 'a'); // 创建key值
msgid = msgget(key, IPC_CREAT|0666); // 创建消息队列
msg.mtype = 1; // 设置消息类型
strcpy(msg.mtext, "Hello, world!"); // 设置消息内容
msgsnd(msgid, &msg, strlen(msg.mtext)+1, 0); // 发送消息
printf("Send message: %s\n", msg.mtext);
exit(0);
}
```
主要函数原型和参数说明:
```c
key_t ftok(const char *pathname, int proj_id); // 创建key值
int msgget(key_t key, int msgflg); // 创建或打开消息队列
// key:消息队列的key值,msgflg:权限标志和标记
ssize_t msgrcv(int msgid, void *msgp, size_t msgsz, long msgtyp, int msgflg); // 接收消息
// msgid:消息队列的ID,msgp:存储消息的缓冲区,msgsz:消息缓冲区的大小,msgtyp:消息类型,msgflg:权限标志和标记
int msgsnd(int msgid, const void *msgp, size_t msgsz, int msgflg); // 发送消息
// msgid:消息队列的ID,msgp:存储消息的缓冲区,msgsz:消息缓冲区的大小,msgflg:权限标志和标记
```
接收消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAXSIZE 128
struct msgbuf
{
long mtype;
char mtext[MAXSIZE];
};
int main()
{
key_t key;
int msgid;
struct msgbuf msg;
key = ftok(".", 'a'); // 创建key值
msgid = msgget(key, IPC_CREAT|0666); // 创建消息队列
msgrcv(msgid, &msg, MAXSIZE, 1, 0); // 接收消息
printf("Receive message: %s\n", msg.mtext);
exit(0);
}
```
主要函数原型和参数说明与发送消息相同。
阅读全文
相关推荐
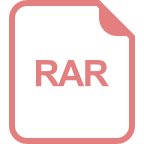
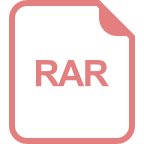
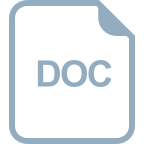
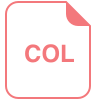


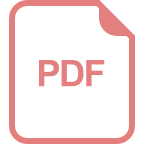
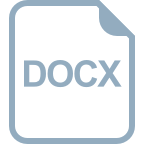
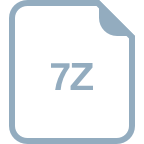
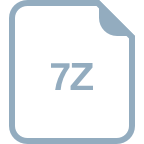
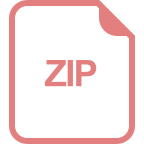