C语言实现消息队列的收发的例子
时间: 2024-02-11 10:04:35 浏览: 69
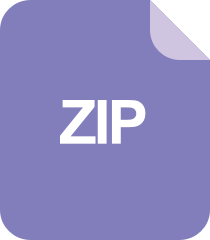
c#消息队列案例
以下是一个使用System V IPC实现消息队列收发的示例程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
// 定义消息结构体
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf msg;
// 生成一个唯一的键值
key = ftok(".", 'q');
if (key == -1) {
perror("ftok");
exit(EXIT_FAILURE);
}
// 创建消息队列
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "Hello, world!");
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 0, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
以下是一个使用POSIX IPC实现消息队列收发的示例程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <mqueue.h>
#define MSG_SIZE 1024
#define QUEUE_NAME "/my_queue"
int main() {
mqd_t mq;
struct mq_attr attr;
char buffer[MSG_SIZE + 1];
int msg_len;
// 设置消息队列的属性
attr.mq_flags = 0;
attr.mq_maxmsg = 10;
attr.mq_msgsize = MSG_SIZE;
attr.mq_curmsgs = 0;
// 创建消息队列
mq = mq_open(QUEUE_NAME, O_CREAT | O_RDWR, 0666, &attr);
if (mq == (mqd_t) -1) {
perror("mq_open");
exit(EXIT_FAILURE);
}
// 发送消息
if (mq_send(mq, "Hello, world!", MSG_SIZE, 0) == -1) {
perror("mq_send");
exit(EXIT_FAILURE);
}
// 接收消息
msg_len = mq_receive(mq, buffer, MSG_SIZE, NULL);
if (msg_len == -1) {
perror("mq_receive");
exit(EXIT_FAILURE);
}
buffer[msg_len] = '\0';
printf("Received message: %s\n", buffer);
// 删除消息队列
if (mq_close(mq) == -1) {
perror("mq_close");
exit(EXIT_FAILURE);
}
if (mq_unlink(QUEUE_NAME) == -1) {
perror("mq_unlink");
exit(EXIT_FAILURE);
}
return 0;
}
```
阅读全文
相关推荐

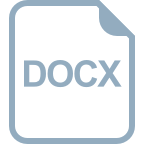
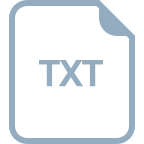
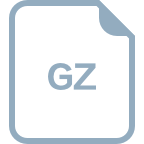
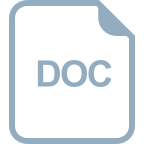
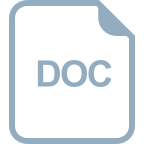


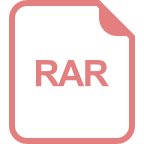
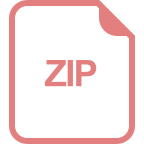
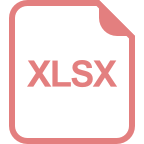