c语言消息队列怎么实现
时间: 2023-03-05 13:31:18 浏览: 104
在 C 语言中,可以通过 System V 消息队列或 POSIX 消息队列来实现消息队列。
对于 System V 消息队列,可以使用以下系统调用来创建和使用消息队列:
1. msgget():创建或访问一个消息队列。
2. msgsnd():向消息队列发送消息。
3. msgrcv():从消息队列中接收消息。
4. msgctl():控制消息队列。
下面是一个使用 System V 消息队列的简单示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
struct message {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
int msqid;
struct message msg;
key_t key;
// 创建消息队列,key 值可以通过 ftok() 函数生成
key = ftok(".", 'a');
if ((msqid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget");
exit(1);
}
// 向消息队列发送消息
msg.mtype = 1;
strcpy(msg.mtext, "Hello, world!");
if (msgsnd(msqid, &msg, sizeof(struct message) - sizeof(long), 0) == -1) {
perror("msgsnd");
exit(1);
}
// 从消息队列接收消息
if (msgrcv(msqid, &msg, sizeof(struct message) - sizeof(long), 0, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message: %s\n", msg.mtext);
// 删除消息队列
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
对于 POSIX 消息队列,可以使用以下系统调用来创建和使用消息队列:
1. mq_open():创建或打开一个消息队列。
2. mq_send():向消息队列发送消息。
3. mq_receive():从消息队列中接收消息。
4. mq_close():关闭消息队列。
5. mq_unlink():删除消息队列。
下面是一个使用 POSIX 消息队列的简单示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <mqueue.h>
#define MSG_SIZE 1024
int main() {
mqd_t mqd;
char *msg = "Hello, world!";
char buf[MSG_SIZE];
struct mq_attr attr = { .mq_maxmsg = 10, .mq_msgsize = MSG_SIZE };
// 创建消息队列
if ((mqd = mq_open("/test", O_CREAT | O_RDWR, 0666, &attr)) == -1) {
perror("mq_open");
exit(1);
}
// 向消息队列发送消息
if (mq_send(mqd, msg, strlen(msg), 0) == -1) {
perror("mq_send");
exit(1);
}
// 从消息队列接收消息
if (mq_receive(m
相关推荐
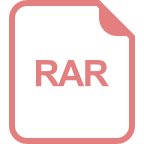














