请帮我写一个,将jar包转换为exe的脚本
时间: 2023-11-27 11:49:40 浏览: 39
好的,你可以使用以下的脚本来将 jar 包转换为 exe 文件:
```python
import os
import sys
import shutil
from subprocess import Popen, PIPE
def run_command(command):
process = Popen(command, stdout=PIPE, stderr=PIPE)
output, error = process.communicate()
return output, error, process.returncode
def create_exe(jar_path, exe_name):
if not os.path.exists(jar_path):
print("Jar file not found!")
return
if sys.platform != "win32":
print("This script only works on Windows!")
return
# Create temporary directory
tmp_dir = "tmp"
if os.path.exists(tmp_dir):
shutil.rmtree(tmp_dir)
os.mkdir(tmp_dir)
# Copy jar file to temporary directory
shutil.copy(jar_path, tmp_dir)
# Create batch file to launch jar file
with open(os.path.join(tmp_dir, "launch.bat"), "w") as f:
f.write("java -jar %s" % os.path.basename(jar_path))
# Convert batch file to exe file
command = ["pyinstaller", "--onefile", "--windowed", "--name=%s" % exe_name, "launch.bat"]
output, error, returncode = run_command(command)
if returncode != 0:
print("Failed to create exe file!")
print(error.decode("utf-8"))
else:
print("Exe file created successfully!")
print("Output file: %s.exe" % exe_name)
# Clean up temporary directory
shutil.rmtree(tmp_dir)
# Example usage
create_exe("myapp.jar", "myapp")
```
这个脚本使用了 PyInstaller 库来将 batch 文件转换为 exe 文件。你需要先安装 PyInstaller 库,可以使用 pip 命令进行安装:
```
pip install pyinstaller
```
使用时,将上面的脚本保存为一个 Python 文件,例如 `convert_jar_to_exe.py`,然后在命令行中运行:
```
python convert_jar_to_exe.py
```
脚本会提示你输入 jar 文件的路径和要创建的 exe 文件的名称。执行完成后,会在同级目录下创建一个 exe 文件。
注意,这个脚本只适用于 Windows 系统。如果你需要在其他操作系统上运行,需要修改脚本中的一些部分。
相关推荐
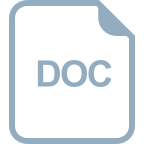
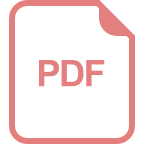
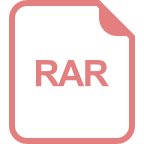














