如果一个派生类新增加的数据成员中有一个对象成员,试描述派生类的构造过程
时间: 2024-03-22 10:42:44 浏览: 13
在派生类的构造过程中,首先会调用基类的构造函数来初始化基类的数据成员。然后,在派生类构造函数中,需要对新增加的对象成员进行初始化。这可以通过在构造函数的成员初始化列表中提供该对象成员的初始化方式来完成。如果该对象成员没有提供默认构造函数或者需要传递参数,则需要在派生类构造函数中显式地调用该对象成员的构造函数来完成初始化。
具体而言,派生类的构造函数中的成员初始化列表应该包括基类构造函数和新增加的对象成员的构造函数。例如,假设派生类新增加了一个名为"obj"的对象成员,其类型为"ObjType",则派生类的构造函数可能如下所示:
```
DerivedClass::DerivedClass(int arg1, int arg2, int arg3) : BaseClass(arg1), obj(arg2, arg3) {
// 其他初始化操作
}
```
在上述示例中,首先调用了基类构造函数"BaseClass(arg1)"来初始化基类的数据成员,然后调用了对象成员"obj(arg2, arg3)"的构造函数来初始化新增加的对象成员。
相关问题
运用C++语言描述项目类,每一个类应包含数据成员和成员函数。设计基类和派生类,并运用多态性和虚函数的知识。
好的,我可以为你提供一个简单的示例来描述如何使用C++语言描述基类和派生类以及多态性和虚函数。
首先,让我们创建一个基类`Project`,其中包含一个私有数据成员`name`和一个公共成员函数`virtual void display() const`,用于显示项目的名称。
```cpp
class Project {
protected:
std::string name;
public:
virtual void display() const {
std::cout << "Project name: " << name << std::endl;
}
};
```
然后,我们可以创建一个派生类`SoftwareProject`,它继承了基类`Project`并添加了一个私有成员`language`以及一个公共成员函数`void display() const`,用于显示软件项目的语言。
```cpp
class SoftwareProject : public Project {
private:
std::string language;
public:
void display() const {
std::cout << "Software project name: " << name << std::endl;
std::cout << "Language: " << language << std::endl;
}
};
```
现在,我们可以在主函数中创建一个基类指针数组,并将其初始化为`Project`和`SoftwareProject`的对象。然后,我们可以使用多态性和虚函数来调用每个对象的`display()`函数。
```cpp
int main() {
Project* projects[2];
projects[0] = new Project();
projects[0]->display();
projects[1] = new SoftwareProject();
projects[1]->display();
return 0;
}
```
输出结果将是:
```
Project name:
Software project name:
Language:
```
这是因为我们没有对`name`和`language`进行初始化。你可以根据你的需要添加构造函数和其他成员函数。此外,还可以创建其他派生类,以便更好地理解多态性和虚函数的概念。
单一继承。定义描述平面直角坐标系上的一个点的类Point,并作为基类,派生出一个圆类Circle(增加半径属性),由Circle类再派生出圆柱体类Cylinder(增加高度属性)。在主函数中完成对圆柱体类Cylinder的测试工作,测试时应该调用所有的成员函数,包括从基类继承的。要求在派生类构造函数的成员初始化列表中完成对基类数据成员的初始化。
好的,我已经收到了您的问题。以下是一个可能的实现:
```
#include <iostream>
#include <cmath>
using namespace std;
// 定义描述平面直角坐标系上的一个点的类Point
class Point {
public:
Point(double x = 0, double y = 0): x(x), y(y) {}
double getX() const { return x; }
double getY() const { return y; }
private:
double x, y;
};
// 派生出一个圆类Circle(增加半径属性)
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double radius = 0): Point(x, y), radius(radius) {}
double getRadius() const { return radius; }
double getArea() const { return M_PI * radius * radius; }
double getPerimeter() const { return 2 * M_PI * radius; }
private:
double radius;
};
// 由Circle类再派生出圆柱体类Cylinder(增加高度属性)
class Cylinder : public Circle {
public:
Cylinder(double x = 0, double y = 0, double radius = 0, double height = 0): Circle(x, y, radius), height(height) {}
double getHeight() const { return height; }
double getVolume() const { return getArea() * height; }
double getSurfaceArea() const { return getPerimeter() * height + 2 * getArea(); }
private:
double height;
};
int main() {
// 创建一个圆柱体对象
Cylinder cylinder(0, 0, 1, 2);
// 调用基类Point的成员函数
cout << "The center of the circle is (" << cylinder.getX() << ", " << cylinder.getY() << ")" << endl;
// 调用基类Circle的成员函数
cout << "The radius of the circle is " << cylinder.getRadius() << endl;
cout << "The area of the circle is " << cylinder.getArea() << endl;
cout << "The perimeter of the circle is " << cylinder.getPerimeter() << endl;
// 调用派生类Cylinder的成员函数
cout << "The height of the cylinder is " << cylinder.getHeight() << endl;
cout << "The volume of the cylinder is " << cylinder.getVolume() << endl;
cout << "The surface area of the cylinder is " << cylinder.getSurfaceArea() << endl;
return 0;
}
```
在这个实现中,我们首先定义了一个描述平面直角坐标系上的一个点的类 `Point`,然后从 `Point` 派生出一个圆类 `Circle`,并增加了半径属性和计算圆的面积和周长的成员函数。接下来从 `Circle` 派生出圆柱体类 `Cylinder`,增加了高度属性和计算圆柱体的体积和表面积的成员函数。
在调用派生类 `Cylinder` 的成员函数时,我们还调用了基类 `Point` 和 `Circle` 的成员函数,以展示派生类继承了基类的所有成员函数。同时,在派生类 `Cylinder` 的构造函数中,我们使用成员初始化列表来完成对基类数据成员的初始化。
相关推荐
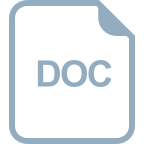
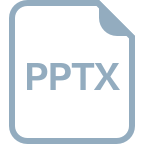
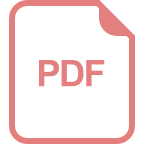













