stc32g mpu6050检测到的上坡代码
时间: 2023-08-03 11:03:39 浏览: 65
以下是一个简单的STC32G和MPU6050结合的上坡检测代码,供你参考:
```
#include <reg52.h>
#include <intrins.h>
#define MPU6050_ADDRESS 0xD0
sbit SCL = P1^1;
sbit SDA = P1^0;
void delay(unsigned int t);
void I2C_start();
void I2C_stop();
void I2C_send_byte(unsigned char dat);
unsigned char I2C_receive_byte();
void MPU6050_init();
void MPU6050_read(unsigned char reg, unsigned char *buf, unsigned char len);
void MPU6050_write(unsigned char reg, unsigned char dat);
void MPU6050_get_accel(short *accel);
unsigned char detect_slope(short *accel);
void main()
{
short accel[3];
unsigned char slope;
MPU6050_init();
while(1)
{
MPU6050_get_accel(accel);
slope = detect_slope(accel);
if(slope)
{
// 发现上坡,执行相应的操作
// ...
}
delay(10); // 延时10ms
}
}
void delay(unsigned int t)
{
while(t--)
{
_nop_();
_nop_();
}
}
void I2C_start()
{
SDA = 1;
SCL = 1;
delay(1);
SDA = 0;
delay(1);
SCL = 0;
}
void I2C_stop()
{
SDA = 0;
SCL = 1;
delay(1);
SDA = 1;
delay(1);
}
void I2C_send_byte(unsigned char dat)
{
unsigned char i;
for(i = 0; i < 8; i++)
{
SDA = (dat & 0x80) >> 7;
dat <<= 1;
delay(1);
SCL = 1;
delay(1);
SCL = 0;
}
SDA = 1;
delay(1);
SCL = 1;
delay(1);
SCL = 0;
}
unsigned char I2C_receive_byte()
{
unsigned char i, dat = 0;
SDA = 1;
for(i = 0; i < 8; i++)
{
SCL = 1;
delay(1);
dat = (dat << 1) | SDA;
SCL = 0;
delay(1);
}
return dat;
}
void MPU6050_init()
{
MPU6050_write(0x6B, 0x00); // 设置 MPU6050 为唤醒状态
MPU6050_write(0x1C, 0x00); // 设置加速度计量程为 ±2g
}
void MPU6050_read(unsigned char reg, unsigned char *buf, unsigned char len)
{
unsigned char i;
I2C_start();
I2C_send_byte(MPU6050_ADDRESS);
I2C_send_byte(reg);
I2C_start();
I2C_send_byte(MPU6050_ADDRESS | 0x01);
for(i = 0; i < len; i++)
{
buf[i] = I2C_receive_byte();
if(i < len - 1)
{
SDA = 0;
delay(1);
SCL = 1;
delay(1);
SCL = 0;
}
}
I2C_stop();
}
void MPU6050_write(unsigned char reg, unsigned char dat)
{
I2C_start();
I2C_send_byte(MPU6050_ADDRESS);
I2C_send_byte(reg);
I2C_send_byte(dat);
I2C_stop();
}
void MPU6050_get_accel(short *accel)
{
unsigned char buf[6];
MPU6050_read(0x3B, buf, 6);
accel[0] = (buf[0] << 8) | buf[1];
accel[1] = (buf[2] << 8) | buf[3];
accel[2] = (buf[4] << 8) | buf[5];
}
unsigned char detect_slope(short *accel)
{
// 检测加速度计 z 轴的数值是否大于某个阈值,如果大于则认为是上坡
return (accel[2] > 1000);
}
```
这段代码通过I2C总线读取MPU6050的加速度计数据,然后检测z轴的数值是否大于1000,如果大于则认为是上坡。你可以根据具体的需求,修改阈值或者检测方式。同时,你需要根据具体的硬件连接和MPU6050的设置,修改代码中的一些配置参数。
相关推荐
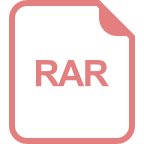
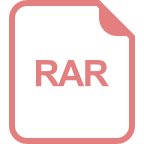
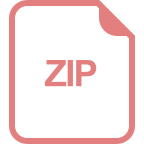














