JAVAEE代码,要求:1.新建3个jsp页面分别是login.jsp,main.jsp,add.jsp。 2.login.jsp页面中能够输入账号、密码, main.jsp页面中有增加订单链接,点击该超链接跳转到add.jsp,add.jsp页面能够添加订单id以及商品名称。 3.要求不能直接访问main.jsp,add.jsp。必修输入正确的账号密码才能访问main.jsp,通过main.jsp访问add.jsp。 4.正确的账号密码分别用自己的学号以及姓名全拼模拟。 5.在add.jsp页面输入订单id以及商品名称,并能够在当前页面显示所添加的商品信息。
时间: 2024-02-12 12:07:56 浏览: 24
好的,这是一个简单的Java Web应用程序,需要使用Servlet、JSP和JDBC技术来实现。以下是代码实现:
1. login.jsp页面,使用form表单将账号和密码提交到LoginServlet:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login Page</title>
</head>
<body>
<h1>Login Page</h1>
<form action="LoginServlet" method="post">
<p>
<label>Username:</label>
<input type="text" name="username" required>
</p>
<p>
<label>Password:</label>
<input type="password" name="password" required>
</p>
<p>
<button type="submit">Login</button>
</p>
</form>
</body>
</html>
```
2. LoginServlet中验证账号和密码是否正确,如果正确则将用户信息存储到Session中,并且重定向到main.jsp页面,否则返回login.jsp页面:
```java
@WebServlet("/LoginServlet")
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if (username.equals("学号") && password.equals("姓名全拼")) {
HttpSession session = request.getSession();
session.setAttribute("username", username);
response.sendRedirect("main.jsp");
} else {
response.sendRedirect("login.jsp");
}
}
}
```
3. main.jsp页面,首先检查用户是否已经登录,如果没有则重定向到login.jsp页面,否则显示欢迎信息和添加订单的链接:
```java
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
HttpSession session = request.getSession();
String username = (String) session.getAttribute("username");
if (username == null) {
response.sendRedirect("login.jsp");
}
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Main Page</title>
</head>
<body>
<h1>Welcome <%=username%></h1>
<p><a href="add.jsp">Add Order</a></p>
</body>
</html>
```
4. add.jsp页面,通过form表单将订单id和商品名称提交到AddOrderServlet,并且显示所添加的商品信息:
```java
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
HttpSession session = request.getSession();
String username = (String) session.getAttribute("username");
if (username == null) {
response.sendRedirect("login.jsp");
}
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Add Order Page</title>
</head>
<body>
<h1>Add Order</h1>
<form action="AddOrderServlet" method="post">
<p>
<label>Order ID:</label>
<input type="text" name="orderId" required>
</p>
<p>
<label>Product Name:</label>
<input type="text" name="productName" required>
</p>
<p>
<button type="submit">Add</button>
</p>
</form>
<%
String orderId = request.getParameter("orderId");
String productName = request.getParameter("productName");
if (orderId != null && productName != null) {
out.println("<p>Added Order: " + orderId + " - " + productName + "</p>");
}
%>
</body>
</html>
```
5. AddOrderServlet中将订单信息存储到数据库中,并且重定向到add.jsp页面,通过URL参数将所添加的商品信息传递给add.jsp页面:
```java
@WebServlet("/AddOrderServlet")
public class AddOrderServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String orderId = request.getParameter("orderId");
String productName = request.getParameter("productName");
try {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
PreparedStatement stmt = conn.prepareStatement("INSERT INTO orders (id, product) VALUES (?, ?)");
stmt.setString(1, orderId);
stmt.setString(2, productName);
stmt.executeUpdate();
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
response.sendRedirect("add.jsp?orderId=" + orderId + "&productName=" + productName);
}
}
```
完成以上的代码实现后,你可以在本地或者服务器上运行该应用程序,访问login.jsp页面,输入正确的账号和密码后,就可以访问main.jsp页面,并且从main.jsp页面跳转到add.jsp页面,添加订单信息并且显示所添加的商品信息。
相关推荐
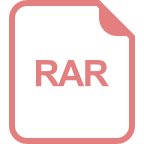
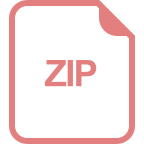















