public void OnDropButtonClick() { int randomNumber = Random.Range(1, 100); if (randomNumber <= 70)//50 { Instantiate(stonePrefab, transform.position, Quaternion.identity); shitou += 30; // 点击按钮1,增加 shitouText.text = "shitou: " + shitou.ToString(); // 更新显示石头的文本框 }
时间: 2024-02-15 07:27:31 浏览: 112
这是一个Unity游戏中的脚本代码,其中包括一个OnDropButtonClick()函数。当按钮被点击时,会生成一个名为stonePrefab的游戏对象,并将其位置设置为当前脚本所附加的对象的位置。接着,它会生成一个1到100之间的随机数,如果随机数小于等于70,就会执行一些代码。具体来说,它会将一个名为shitou的变量增加30,并在游戏界面上更新一个名为shitouText的文本框,显示shitou的值。
相关问题
public void OnDropButtonClick() { int randomNumber = Random.Range(1, 101); if (randomNumber <= 40) { Instantiate(stonePrefab, transform.position, Quaternion.identity); shitou += 30; // 点击按钮1,增加 shitouText.text = "shitou: " + shitou.ToString(); // 更新显示石头的文本框 } else if (randomNumber <= 70) { Instantiate(woodPrefab, transform.position, Quaternion.identity); mutou += 50; // 点击按钮1,增加 mutouText.text = "mutou: " + mutou.ToString(); // 更新显示木头的文本框 }
这段代码看起来像是Unity引擎的C#脚本,其中包含一个名为OnDropButtonClick的公共方法。当点击某个按钮时,此方法将被调用。在方法的第一行,定义了一个名为randomNumber的整数变量,使用Random类的Range方法生成一个1到100之间(包括1和100)的随机整数。if语句检查randomNumber是否小于或等于40,如果是,则在场景中生成一个名为stonePrefab的游戏对象,并将该对象的位置设置为脚本所属的游戏对象的位置。此外,还会增加一个名为shitou的整数变量的值,并将其更新到一个名为shitouText的UI文本框中。
如果randomNumber不小于40,那么会执行else if块。在这个块中,代码生成一个名为woodPrefab的游戏对象,并将其位置设置为脚本所属的游戏对象的位置。还会增加一个名为mutou的整数变量的值,并将其更新到一个名为mutouText的UI文本框中。这个else if块只会在randomNumber大于40且小于或等于70的情况下被执行。
如果randomNumber大于70,那么什么也不会发生,因为没有else块来处理这种情况。这段代码可能用于一个点击按钮的事件处理程序,每次点击按钮时都会执行该代码。
2.Reliability and Maintainability Simulation involves a large number of random variables. The use of dynamic arrays will greatly improve the efficiency of simulation and the scale of problem solving. Please design Vector. This problem requires the implementation of a vector class template, which can realize the storage and access of data. (1) [] operator can only access the existing elements. (2) The add method can automatically expand the internal storage space when accessing. Note that the behavior of this vector is different from that of std:: vector. Function interface definition: template <class T> class Vector { ... } Example of referee test procedure: #include <iostream> using namespace std; /* Todo: write down your code here! */ int main() { Vector<int> vint; int n,m; cin >> n >> m; for ( int i=0; i<n; i++ ) { // add() can inflate the vector automatically vint.add(i); } // get_size() returns the number of elements stored in the vector cout << vint.get_size() << endl; cout << vint[m] << endl; // remove() removes the element at the index which begins from zero vint.remove(m); cout << vint.add(-1) << endl; cout << vint[m] << endl; Vector<int> vv = vint; cout << vv[vv.get_size()-1] << endl; vv.add(m); cout << vint.get_size() << endl; } Input example: 100 50 Output example: 100 50 99 51 -1 100
Here is the implementation of the Vector class template:
```
template<class T>
class Vector {
private:
T* data; // pointer to the actual data
int capacity; // current capacity of the vector
int size; // current size of the vector
public:
// constructor
Vector() {
data = nullptr;
capacity = 0;
size = 0;
}
// copy constructor
Vector(const Vector& other) {
capacity = other.capacity;
size = other.size;
data = new T[capacity];
for (int i = 0; i < size; i++) {
data[i] = other.data[i];
}
}
// destructor
~Vector() {
if (data != nullptr) {
delete[] data;
}
}
// [] operator for accessing elements
T& operator[](int index) {
if (index >= size) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
// add method for adding elements
void add(const T& value) {
if (size == capacity) {
int newCapacity = capacity == 0 ? 1 : capacity * 2;
T* newData = new T[newCapacity];
for (int i = 0; i < size; i++) {
newData[i] = data[i];
}
if (data != nullptr) {
delete[] data;
}
data = newData;
capacity = newCapacity;
}
data[size++] = value;
}
// remove method for removing elements
void remove(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
for (int i = index; i < size - 1; i++) {
data[i] = data[i + 1];
}
size--;
}
// get_size method for getting the current size of the vector
int get_size() const {
return size;
}
};
```
In the main function, we first create a Vector object `vint` of type `int`. We read in two integers `n` and `m` from the input, and then use a for loop to add `n` elements to the vector using the `add` method. We then print the size of the vector using the `get_size` method, and print the element at index `m` using the `[]` operator. We then remove the element at index `m` using the `remove` method, and add a new element with value `-1` using the `add` method. We print the new element at index `m` using the `[]` operator. We create a new Vector object `vv` by copying `vint`, and print the last element of `vv` using the `[]` operator. Finally, we add an element with value `m` to `vv`, and print the size of `vint` using the `get_size` method.
I hope this helps!
阅读全文
相关推荐
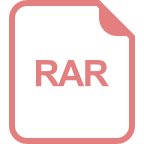
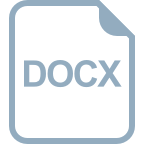
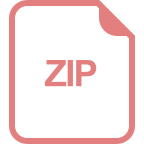
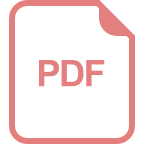
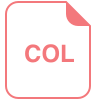











