编写c程序: 用fork创建子进程,之后父进程调用系统调用kill()向子进程发送信号,子进程获得信号后终止并输出终止信息,父进程等子进程终止后终止并输出信息 Parent process is killed。
时间: 2024-10-18 11:08:42 浏览: 35
在C语言中,你可以使用`fork()`函数创建一个新的子进程,并通过`kill()`函数向子进程发送信号。下面是一个简单的示例,演示如何实现这个过程:
首先,你需要包含必要的头文件:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <signal.h>
```
然后,设置一个信号处理器(在这里我们只处理SIGINT信号,例如用户按下Ctrl+C):
```c
void signal_handler(int signum) {
if (signum == SIGINT) {
printf("Parent process received SIGINT and will terminate.\n");
_exit(0); // 父进程退出
}
}
int main() {
// 注册信号处理器
struct sigaction action;
action.sa_handler = signal_handler;
sigemptyset(&action.sa_mask);
action.sa_flags = 0;
if (sigaction(SIGINT, &action, NULL) == -1) {
perror("Failed to set signal handler");
return 1;
}
pid_t child_pid = fork(); // 创建子进程
if (child_pid == 0) { // 子进程
printf("Child process started. Waiting for parent's signal...\n");
// 在这里添加接收信号的循环,直到接收到信号或达到其他条件
while (true) {
// ... (省略接收信号的部分)
}
printf("Child process terminated by parent's signal.\n");
_exit(0); // 子进程退出
} else if (child_pid > 0) { // 父进程
printf("Parent process (%d) created child process (%d)\n", getpid(), child_pid);
// 发送信号给子进程
kill(child_pid, SIGINT); // SIGINT通常表示中断请求
// 等待子进程结束
wait(NULL); // 父进程等待子进程完成
printf("Parent process finished after child process termination.\n");
} else { // fork失败的情况
perror("Fork failed");
return 1;
}
return 0; // 结束主函数
}
```
在这个示例中,当父进程接收到SIGINT信号时,它会发送同样的信号给子进程。子进程收到信号后会停止执行并输出相关信息,最后父进程会在子进程结束后终止。
阅读全文
相关推荐
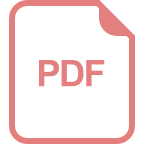
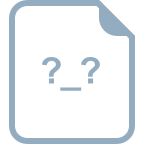
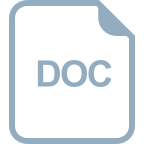





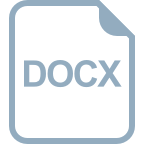
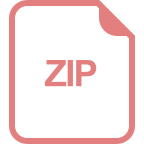
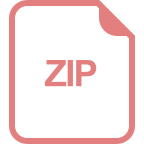