实现一个圆柱体类,类中包含私有数据成员半径和高;包含设置圆柱体半径和高、计算圆柱体体积;获取圆柱体半径、高、圆柱体体积的成员函数;能够对数据有效性进行判断处理。编写主函数测试类的使用。使用C++实现代码
时间: 2024-12-22 17:13:22 浏览: 14
```cpp
#include <iostream>
#include <cmath> // 引入math库用于计算圆周率π
class Cylinder {
private:
double radius; // 圆柱体半径
double height; // 圆柱体高
const static double PI = 3.14159; // 定义圆周率常量
public:
// 构造函数,设置初始值并验证数据有效性
Cylinder(double r, double h) : radius(r), height(h) {
if (r <= 0 || h <= 0) {
throw std::invalid_argument("Radius and height must be positive.");
}
}
// 设置圆柱体半径和高的成员函数
void setRadiusAndHeight(double new_radius, double new_height) {
if (new_radius <= 0 || new_height <= 0) {
throw std::invalid_argument("New radius and height must be positive.");
}
radius = new_radius;
height = new_height;
}
// 计算圆柱体体积的成员函数
double calculateVolume() {
return PI * pow(radius, 2) * height;
}
// 获取圆柱体半径、高、体积的成员函数
double getRadius() const { return radius; }
double getHeight() const { return height; }
double getVolume() const { return calculateVolume(); }
};
int main() {
try {
// 创建一个圆柱体实例
Cylinder cylinder(3.5, 7);
// 测试成员函数
std::cout << "Initial volume: " << cylinder.getVolume() << std::endl;
// 修改圆柱体尺寸并重新计算体积
cylinder.setRadiusAndHeight(4.0, 8.0);
std::cout << "New volume: " << cylinder.getVolume() << std::endl;
// 试图设置无效数据,会抛出异常
// cylinder.setRadiusAndHeight(-1, 10); // 这里会引发异常
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
这个代码定义了一个`Cylinder`类,包含了基本的数据成员和相应的操作。通过构造函数、设置方法和计算方法,实现了圆柱体的创建、修改以及体积的计算。当尝试设置无效数据时,会抛出异常。
阅读全文
相关推荐
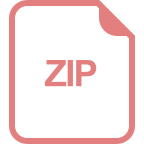
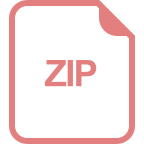
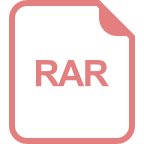
















