js es12 常用代码段
时间: 2023-02-14 15:26:21 浏览: 67
JavaScript ES12 中常用的代码段包括:
1. 利用 Object.fromEntries() 方法将键值对数组转化为对象。
```js
const entries = [['a', 1], ['b', 2], ['c', 3]];
const obj = Object.fromEntries(entries);
console.log(obj); // { a: 1, b: 2, c: 3 }
```
2. 利用 String.prototype.matchAll() 方法获取字符串中所有匹配的子串。
```js
const str = 'hello world';
const regex = /l/g;
const matches = [...str.matchAll(regex)];
console.log(matches); // [["l", index: 2, input: "hello world"], ["l", index: 3, input: "hello world"], ["l", index: 9, input: "hello world"]]
```
3. 利用 Array.prototype.flat() 方法将多维数组扁平化。
```js
const arr = [1, 2, [3, 4, [5, 6]]];
const flatArr = arr.flat(Infinity);
console.log(flatArr); // [1, 2, 3, 4, 5, 6]
```
4. 利用 Promise.allSettled() 方法获取所有 Promise 的状态。
```js
const p1 = Promise.resolve(1);
const p2 = Promise.reject(2);
const p3 = Promise.resolve(3);
const results = await Promise.allSettled([p1, p2, p3]);
console.log(results); // [{status: "fulfilled", value: 1}, {status: "rejected", reason: 2}, {status: "fulfilled", value: 3}]
```
5. 利用 Optional Chaining 操作符 ?. 来避免链式调用时的 null 问题。
```js
const obj = { a: { b: { c: 1 } } };
const value = obj?.a?.b?.c;
console.log(value); // 1
```
这只是 ES12 中常用的代码段之一,更多详细信息可以参考官方文档。
相关推荐
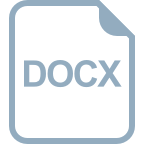
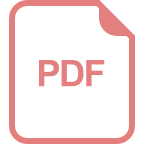
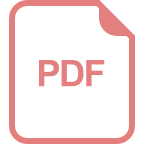














