SSM框架中使用的设计模式及具体代码
时间: 2024-02-16 17:04:26 浏览: 36
SSM框架中使用的设计模式有:
1. MVC模式:SSM框架采用了MVC模式,即将应用程序分为三个部分:模型(Model)、视图(View)和控制器(Controller)。在SSM框架中,Model一般指的是MyBatis中的Mapper接口以及对应的Mapper.xml文件,View一般指的是前端页面,Controller一般指的是Spring MVC中的Controller类。
2. 工厂模式:Spring框架中的Bean工厂就是一个典型的工厂模式的应用。在SSM框架中,我们可以通过Spring框架来创建对象,并且通过IoC/DI的方式来管理这些对象的生命周期。
3. 单例模式:Spring框架中的Bean默认是单例模式的,即在容器中只会存在一个实例。这样可以避免频繁的创建和销毁对象,提高系统性能。
4. 代理模式:MyBatis中的Mapper接口是一个代理接口,其实现类由MyBatis框架来生成。在SSM框架中,我们可以通过Spring AOP来实现代理模式,从而实现事务管理、日志记录等功能。
具体代码示例:
1. MVC模式
Controller类:
```java
@Controller
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@RequestMapping("/list")
public String list(Model model) {
List<User> userList = userService.getUserList();
model.addAttribute("userList", userList);
return "user/list";
}
}
Model类:
public class User {
private Integer id;
private String username;
private String password;
// getter和setter方法省略
}
Mapper接口:
public interface UserMapper {
List<User> selectAll();
}
Mapper.xml文件:
<select id="selectAll" resultType="com.example.model.User">
select id, username, password from user
</select>
```
2. 工厂模式
Bean配置文件:
```xml
<bean id="userService" class="com.example.service.impl.UserServiceImpl">
<property name="userDao" ref="userDao"></property>
</bean>
<bean id="userDao" class="com.example.dao.impl.UserDaoImpl">
<property name="dataSource" ref="dataSource"></property>
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/test"></property>
<property name="username" value="root"></property>
<property name="password" value="123456"></property>
</bean>
```
3. 单例模式
Bean配置文件:
```xml
<bean id="userService" class="com.example.service.impl.UserServiceImpl" scope="singleton">
<property name="userDao" ref="userDao"></property>
</bean>
<bean id="userDao" class="com.example.dao.impl.UserDaoImpl" scope="singleton">
<property name="dataSource" ref="dataSource"></property>
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource" scope="singleton">
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/test"></property>
<property name="username" value="root"></property>
<property name="password" value="123456"></property>
</bean>
```
4. 代理模式
Bean配置文件:
```xml
<bean id="userService" class="com.example.service.impl.UserServiceImpl">
<property name="userDao" ref="userDao"></property>
</bean>
<bean id="userDao" class="com.example.dao.impl.UserDaoImpl">
<property name="dataSource" ref="dataSource"></property>
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/test"></property>
<property name="username" value="root"></property>
<property name="password" value="123456"></property>
</bean>
<aop:config>
<aop:pointcut id="txPointcut" expression="execution(* com.example.service.*.*(..))"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="txPointcut"/>
</aop:config>
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<tx:method name="*" propagation="REQUIRED"/>
</tx:attributes>
</tx:advice>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
```
相关推荐
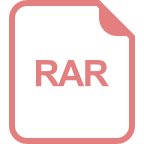
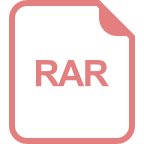
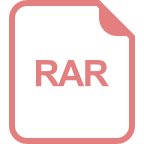














