import tensorflow as tf import matplotlib.pyplot as plt img1 = plt.imread("Trump.jpg")/255. plt.imshow(img1) img2 = plt.imread('Trump2.jpg')/255. plt.imshow(img2) img1 = tf.image.resize(img1,(500,500)) img1 = tf.convert_to_tensor(img1,dtype=tf.float32) # 增加batch维度 img1 = tf.expand_dims(img1,axis=0) img2 = tf.image.resize(img2,(500,500)) img2 = tf.convert_to_tensor(img2,dtype=tf.float32) # 增加batch维度 img2 = tf.expand_dims(img2,axis=0) img = tf.concat([img1,img2],axis=0) roi = tf.image.crop_and_resize(img,[[0.5,0.5,1,1],[0.5,0.5,1,1]],[0,1],crop_size=(100,100)) # 图像展示 plt.imshow(img[0]) plt.imshow(img[1]) plt.imshow(roi[0]) plt.imshow(roi)以上代码有什么问题
时间: 2023-08-31 22:11:19 浏览: 43
以上代码有如下问题:
1. 缺少必要的导入语句,需要在代码开头添加导入tensorflow和matplotlib.pyplot的语句。
2. 在使用plt.imshow函数展示图像时,应该使用不同的子图来展示不同的图像,否则会在同一张图上叠加多个图像。
3. 在使用tf.image.crop_and_resize函数时,boxes参数应该是一个二维张量,每一行代表一个目标框的坐标。而在代码中,boxes参数被赋值为了一个列表,需要将其改为二维张量形式。
4. 在使用tf.image.crop_and_resize函数时,boxes参数和box_indices参数应该有相同的行数,代表每个目标框对应的图像在输入张量中的索引。在代码中,box_indices参数被赋值为了[0,1],但是boxes参数只有一行,需要将其改为两行,以对应两个目标框。
5. 在使用plt.imshow函数展示裁剪后的图像时,需要指定cmap参数为None,否则图像会以灰度图的形式展示。
以下是修改后的代码:
```
import tensorflow as tf
import matplotlib.pyplot as plt
# 加载图像
img1 = plt.imread("Trump.jpg")/255.
img2 = plt.imread('Trump2.jpg')/255.
# 展示图像
plt.subplot(1, 2, 1)
plt.imshow(img1)
plt.subplot(1, 2, 2)
plt.imshow(img2)
plt.show()
# 预处理图像
img1 = tf.image.resize(img1, (500, 500))
img1 = tf.convert_to_tensor(img1, dtype=tf.float32)
img1 = tf.expand_dims(img1, axis=0)
img2 = tf.image.resize(img2, (500, 500))
img2 = tf.convert_to_tensor(img2, dtype=tf.float32)
img2 = tf.expand_dims(img2, axis=0)
img = tf.concat([img1, img2], axis=0)
# 裁剪图像
boxes = tf.constant([[0.5, 0.5, 1, 1], [0.5, 0.5, 1, 1]])
box_indices = tf.constant([0, 1])
roi = tf.image.crop_and_resize(img, boxes, box_indices, crop_size=(100, 100))
# 展示图像
plt.subplot(1, 3, 1)
plt.imshow(img1[0])
plt.subplot(1, 3, 2)
plt.imshow(img2[0])
plt.subplot(1, 3, 3)
plt.imshow(roi[0], cmap=None)
plt.show()
```
相关推荐
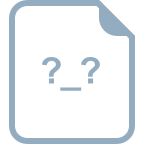
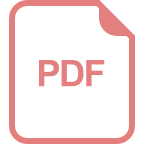
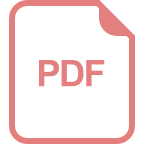














