使用C++,编写一个程序模拟堆栈,要求能够模拟、入栈、出栈、返回栈顶元素等基本操作。栈中元素可用整数代替。不能使用C++模板库预定义的类型。程序运行中可输入多组入栈、出栈操作,每次操作后展示栈中元素。
时间: 2023-12-31 19:03:28 浏览: 25
好的,以下是符合要求的C++代码实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
class Stack {
private:
vector<int> stack;
public:
void push(int item) {
stack.push_back(item);
}
int pop() {
if (!is_empty()) {
int item = stack.back();
stack.pop_back();
return item;
} else {
return -1; // 返回 -1 代表栈为空
}
}
int peek() {
if (!is_empty()) {
return stack.back();
} else {
return -1; // 返回 -1 代表栈为空
}
}
bool is_empty() {
return stack.empty();
}
void print_stack() {
cout << "Stack: ";
for (int item : stack) {
cout << item << " ";
}
cout << endl;
}
};
int main() {
Stack my_stack;
while (true) {
cout << "Please input your operation (push/pop/peek/print/quit):" << endl;
string op;
cin >> op;
if (op == "push") {
cout << "Please input the number you want to push:" << endl;
int num;
cin >> num;
my_stack.push(num);
my_stack.print_stack();
} else if (op == "pop") {
int num = my_stack.pop();
if (num != -1) {
cout << "Pop number: " << num << endl;
}
my_stack.print_stack();
} else if (op == "peek") {
int num = my_stack.peek();
if (num != -1) {
cout << "Peek number: " << num << endl;
}
my_stack.print_stack();
} else if (op == "print") {
my_stack.print_stack();
} else if (op == "quit") {
break;
} else {
cout << "Invalid operation!" << endl;
}
}
return 0;
}
```
这个堆栈程序与 Python 版本实现类似,也实现了基本的入栈、出栈、返回栈顶元素和展示栈中元素的操作。当程序运行时,每次输入一个操作指令,程序会相应地进行操作并展示当前栈中的元素。如果输入 quit,则程序会结束。
相关推荐
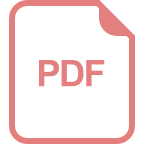
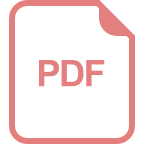








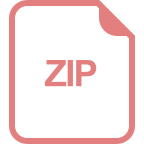
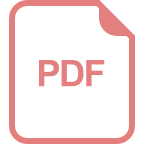
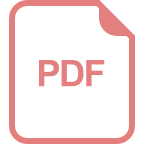
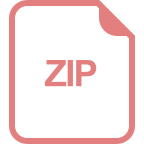
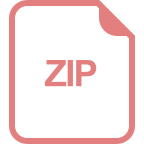
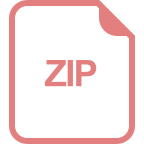
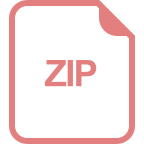