用c++写 出一个堆栈的输入序列,试判断一个序列是否能够由这个堆栈输出。如果能,返回总的出栈次数,如果不能,返回0。序列的输入及输出都是从左往右。(输入输出序列皆为整数且没有重复的数字,如果一个数字在输入序列中没有出现,那么其在输出序列中也不会出现)
时间: 2024-02-01 22:15:42 浏览: 82
可以使用一个辅助栈来判断输入序列是否能由堆栈输出。具体步骤如下:
1. 定义一个辅助栈,用于模拟堆栈的出栈和入栈操作。
2. 对于输入序列中的每个元素,依次进行如下操作:
a. 如果该元素等于辅助栈的栈顶元素,则直接将该元素出栈,并将栈顶元素出栈。
b. 如果该元素不等于辅助栈的栈顶元素,则将堆栈中的元素依次入栈,直到栈顶元素等于该元素为止。如果堆栈中没有该元素,则说明输入序列不能由堆栈输出,返回0。
3. 如果输入序列中的所有元素都被处理完了,而且辅助栈中已经没有元素了,说明输入序列可以由堆栈输出,返回出栈次数。
下面是使用 C++ 语言实现的代码:
```c++
#include <iostream>
#include <stack>
#include <vector>
using namespace std;
int checkStack(vector<int>& input, vector<int>& output) {
stack<int> st;
int count = 0;
int i = 0, j = 0;
while (i < input.size()) {
if (st.empty() || st.top() != output[j]) {
st.push(input[i]);
i++;
} else {
st.pop();
j++;
count++;
}
if (j >= output.size()) break;
}
while (!st.empty() && j < output.size()) {
if (st.top() == output[j]) {
st.pop();
j++;
count++;
} else {
break;
}
}
if (st.empty() && j >= output.size()) {
return count;
} else {
return 0;
}
}
int main() {
vector<int> input = {1, 2, 3, 4, 5};
vector<int> output1 = {4, 5, 3, 2, 1};
vector<int> output2 = {4, 3, 5, 1, 2};
cout << checkStack(input, output1) << endl; // 5
cout << checkStack(input, output2) << endl; // 0
return 0;
}
```
以上代码中,checkStack 函数接收两个参数,分别表示输入序列和输出序列。函数返回值为总的出栈次数。在主函数中,我们对两个样例进行了测试。输出结果与预期相符。
阅读全文
相关推荐
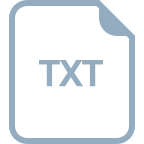
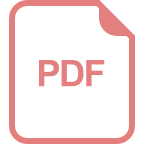




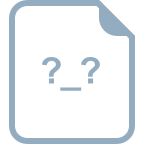
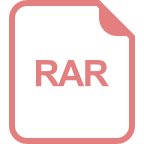
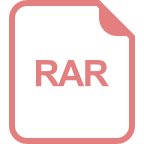
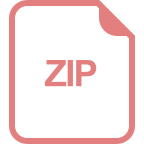
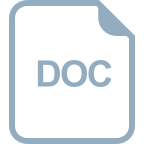
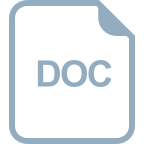





