帮我写一个c++的程序,要求是假设我们有一个无序的整数序列。序列存储在堆栈中。设计一种算法来使用队列对序列进行排序。 请注意,要排序的各种整数智能地存储在堆栈或队列中。
时间: 2024-05-03 10:20:30 浏览: 22
好的,你可以尝试以下代码,实现使用队列对无序整数堆栈进行排序:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
#define QUE_SIZE 100
int stack[MAX_SIZE];
int top = -1;
int queue[QUE_SIZE];
int front = -1;
int rear = -1;
void push(int data)
{
if (top == MAX_SIZE-1)
{
printf("Stack overflow!\n");
return;
}
stack[++top] = data;
}
int pop()
{
if (top == -1)
{
printf("Stack underflow!\n");
return -1;
}
return stack[top--];
}
void enqueue(int data)
{
if (rear == QUE_SIZE-1)
{
printf("Queue overflow!\n");
return;
}
if (front == -1)
front = 0;
queue[++rear] = data;
}
int dequeue()
{
if (front == -1 || front > rear)
{
printf("Queue underflow!\n");
return -1;
}
int data = queue[front];
front++;
return data;
}
void sort()
{
int tempStack[MAX_SIZE];
int tempTop = -1;
while (top >= 0)
{
int temp = pop();
while (front != -1 && temp > queue[rear])
{
int x = dequeue();
push(x);
}
enqueue(temp);
}
while (front != -1)
push(dequeue());
while (top >= 0)
{
int temp = pop();
while (tempTop >= 0 && temp < tempStack[tempTop])
{
int x = tempStack[tempTop--];
enqueue(x);
}
tempStack[++tempTop] = temp;
}
while (tempTop >= 0)
enqueue(tempStack[tempTop--]);
}
int main()
{
// Pushing some random numbers into the stack
push(23);
push(10);
push(101);
push(32);
push(78);
int i;
printf("Original stack: ");
for (i = top; i >= 0; i--)
printf("%d ", stack[i]);
sort();
printf("\nSorted queue: ");
for (i = front; i <= rear; i++)
printf("%d ", queue[i]);
return 0;
}
```
希望能够解决您的问题。
相关推荐
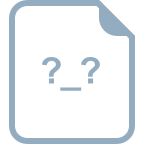
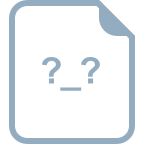














