num_classes = 10 # 类别数量 one_hot_labels = to_categorical(labels, num_classes) # 训练模型 model.fit(x_train, one_hot_labels, epochs=10, batch_size=64) 其中,labels 是原始标签的数组,num_classes 是类别数量原始标签数组指什么?
时间: 2024-03-18 16:43:57 浏览: 262
原始标签数组指的是每个样本对应的真实标签,例如对于手写数字识别任务,原始标签就是每个手写数字所代表的数字(0-9)。在这段代码中,通过将原始标签数组转化为 one-hot 编码的形式,使得模型能够更加容易地理解和处理标签信息。num_classes 则是指总共有多少个不同的类别,在手写数字识别任务中,num_classes 就是10,因为总共有10个数字需要识别。
相关问题
# 创建卷积神经网络模型 model = Sequential() num_classes = 43 # 类别数量 # 添加3个卷积层Conv2D和池化层MaxPooling2D,2个全连接层Dense model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3))) model.add(MaxPooling2D((2, 2))) model.add(Conv2D(64, (3, 3), activation='relu')) model.add(MaxPooling2D((2, 2))) model.add(Conv2D(64, (3, 3), activation='relu')) # 添加全连接层 model.add(Flatten()) model.add(Dense(64, activation='relu')) model.add(Dense(num_classes, activation='softmax'))#输出层使用 softmax 激活函数,输出每个类别的概率 one_hot_labels = to_categorical(y_train, num_classes) # 编译模型 #损失函数为 categorical_crossentropy,用于多类分类问题。 #优化器为 rmsprop。在使用该模型进行训练时,需要将标签转换成 one-hot 编码的形式,可以使用 Keras 中的 to_categorical() 函数来实现。 ##RMSprop 优化器来优化损失函数(categorical_crossentropy) ##用准确率(accuracy)作为评估指标 model.compile(optimizer='rmsprop', loss='categorical_crossentropy', metrics=['accuracy']) # 训练模型 model.fit(X_train, one_hot_labels, epochs=10, batch_size=64)对该模型用测试集进行预测,并获得模型准确率
在模型训练完成后,可以使用测试集数据对模型进行评估,可以使用以下代码来进行预测并计算模型在测试集上的准确率:
```
# 对测试集进行预测
y_pred = model.predict(X_test)
# 将预测结果转换成类别标签
y_pred_classes = np.argmax(y_pred, axis=1)
# 计算模型在测试集上的准确率
accuracy = accuracy_score(y_test, y_pred_classes)
print('Test accuracy:', accuracy)
```
其中,`y_pred`是模型对测试集数据的预测结果,`y_pred_classes`是将预测结果转换成类别标签后的结果,`accuracy_score`是sklearn库中的函数,用于计算预测准确率,`y_test`是测试集数据的真实标签。最后输出的`accuracy`即为模型在测试集上的准确率。
def load_data(): # 加载数据集 with open('D:/浏览器下载/cifar-100-python/cifar-100-python/train', 'rb') as f: data_dict = pickle.load(f, encoding='bytes') x_train = data_dict[b'data'].reshape(-1, 3, 32, 32).transpose(0, 2, 3, 1) y_train = data_dict[b'fine_labels'] with open('D:/浏览器下载/cifar-100-python/cifar-100-python/test', 'rb') as f: data_dict = pickle.load(f, encoding='bytes') x_test = data_dict[b'data'].reshape(-1, 3, 32, 32).transpose(0, 2, 3, 1) y_test = data_dict[b'fine_labels'] # 归一化图像数据 x_train = x_train / 255.0 x_test = x_test / 255.0 # 将标签进行one-hot编码 num_classes = 100 y_train = to_categorical(y_train, num_classes) y_test = to_categorical(y_test, num_classes) return x_train, y_train, x_test, y_test # 加载数据 x_train, y_train, x_test, y_test = load_data() # 数据增强 train_datagen = ImageDataGenerator( rotation_range=20, horizontal_flip=True, zoom_range=0.2, width_shift_range=0.2, height_shift_range=0.2, shear_range=0.2, fill_mode='nearest') train_datagen.fit(x_train) # 增加回调函数 callbacks = [ EarlyStopping(monitor='val_loss', patience=10, verbose=1), ReduceLROnPlateau(monitor='val_loss', factor=0.1, patience=5, verbose=1) ] 请在此代码基础上使用tensorflow2.0版本创建神经网络,并给出最终代码
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Dropout, Flatten, Dense
# 加载数据集
def load_data():
with open('D:/浏览器下载/cifar-100-python/cifar-100-python/train', 'rb') as f:
data_dict = pickle.load(f, encoding='bytes')
x_train = data_dict[b'data'].reshape(-1, 3, 32, 32).transpose(0, 2, 3, 1)
y_train = data_dict[b'fine_labels']
with open('D:/浏览器下载/cifar-100-python/cifar-100-python/test', 'rb') as f:
data_dict = pickle.load(f, encoding='bytes')
x_test = data_dict[b'data'].reshape(-1, 3, 32, 32).transpose(0, 2, 3, 1)
y_test = data_dict[b'fine_labels']
# 归一化图像数据
x_train = x_train / 255.0
x_test = x_test / 255.0
# 将标签进行one-hot编码
num_classes = 100
y_train = tf.keras.utils.to_categorical(y_train, num_classes)
y_test = tf.keras.utils.to_categorical(y_test, num_classes)
return x_train, y_train, x_test, y_test
# 创建神经网络
def create_model():
model = Sequential()
# 卷积层
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', padding='same', input_shape=(32, 32, 3)))
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Conv2D(64, kernel_size=(3, 3), activation='relu', padding='same'))
model.add(Conv2D(64, kernel_size=(3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
# 全连接层
model.add(Flatten())
model.add(Dense(512, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
return model
# 加载数据
x_train, y_train, x_test, y_test = load_data()
# 数据增强
train_datagen = tf.keras.preprocessing.image.ImageDataGenerator(
rotation_range=20,
horizontal_flip=True,
zoom_range=0.2,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
fill_mode='nearest'
)
train_datagen.fit(x_train)
# 增加回调函数
callbacks = [
tf.keras.callbacks.EarlyStopping(monitor='val_loss', patience=10, verbose=1),
tf.keras.callbacks.ReduceLROnPlateau(monitor='val_loss', factor=0.1, patience=5, verbose=1)
]
# 创建模型
model = create_model()
# 编译模型
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
history = model.fit_generator(train_datagen.flow(x_train, y_train, batch_size=64),
epochs=100,
verbose=1,
validation_data=(x_test, y_test),
callbacks=callbacks)
# 评估模型
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
阅读全文
相关推荐
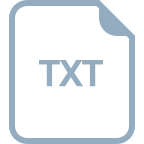
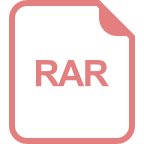
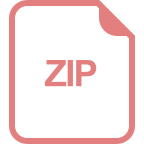

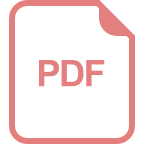
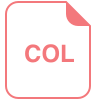
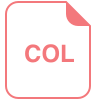









