Function load_earth()
时间: 2024-09-18 16:01:01 浏览: 30
`load_earth()` 函数看起来像是某个编程上下文中用于加载地球数据、地图或者其他地理相关的资源的函数名。这个函数可能是从某种GIS(地理信息系统)库、游戏引擎,或者是数据分析工具中定义的。它的作用可能是从文件、网络或其他数据源获取地球的数据模型、地图图片、地形信息等,并将这些内容加载到内存中,以便后续的处理、分析或显示。
举个例子,如果你正在编写一个Python的地学应用,可能会有这样的函数:
```python
def load_earth(file_path):
from geopandas import read_file # 假设我们使用geopandas读取数据
earth_data = read_file(file_path) # 加载指定路径的ESRI Shapefile
return earth_data
# 使用示例
loaded_earth = load_earth('path/to/world_countries.shp')
```
在这个例子中,`load_earth`函数接受一个参数,即地球数据文件的路径,然后返回一个GeoDataFrame对象。
相关问题
// Load Sentinel-2 TOA reflectance data. var sentinel = ee.ImageCollection('COPERNICUS/S2') .filterDate('2019-01-01', '2019-12-31') .filterBounds(table) .map(function(image) { var cloud_mask = ee.Image(0).where( image.select('QA60').bitwiseAnd(1<<10), 1).rename('cloud_mask'); var cloud_probability = image.select('QA60').bitwiseAnd(1024).rightShift(10).rename('cloud_probability'); var cloud_shadow_probability = image.select('QA60').bitwiseAnd(2048).rightShift(11).rename('cloud_shadow_probability'); var cloud_mask_combined = cloud_mask.or(cloud_probability.gt(20)).or(cloud_shadow_probability.gt(20)); return image.addBands(cloud_mask_combined); }) .map(function(image) { return image.clip(table); }); // Function to mask clouds using the Sentinel-2 cloud mask. var maskClouds = function(image) { var cloudMask = image.select('cloud_mask').not(); return image.updateMask(cloudMask); }; // Function to calculate the NDVI. var calculateNDVI = function(image) { var ndvi = image.normalizedDifference(['B8', 'B4']).rename('ndvi'); return image.addBands(ndvi); }; // Function to calculate the EVI. var calculateEVI = function(image) { var evi = image.expression( '2.5 * (nir - red) / (nir + 6 * red - 7.5 * blue + 1)', { 'nir': image.select('B8'), 'red': image.select('B4'), 'blue': image.select('B2') }).rename('evi'); return image.addBands(evi); }; // Apply the cloud mask, calculate the NDVI and EVI, and combine the bands. var sentinel_ndvi_evi = sentinel .map(maskClouds) .map(calculateNDVI) .map(calculateEVI) .select(['B2', 'B3', 'B4', 'B8', 'ndvi', 'evi']); // Function to filter images based on the quality of the NDVI and EVI. var filterQuality = function(image) { var ndvi_quality = image.select('ndvi').qualityMosaic('ndvi').gte(0.6); var evi_quality = image.select('evi').qualityMosaic('evi').gte(0.6); return image.updateMask(ndvi_quality.and(evi_quality)); }; // Filter the images based on the quality of the NDVI and EVI. var sentinel_filtered = sentinel_ndvi_evi.filter(filterQuality); // Create a median composite of the filtered images and display it. var sentinel_median = sentinel_filtered.median(); Map.addLayer(sentinel_median, {bands: ['B4', 'B3', 'B2'], min: 0, max: 0.3}, 'Sentinel-2 Median Composite');
这段代码使用 Google Earth Engine 平台加载 Sentinel-2 的 TOA 反射率数据,并对其进行云掩膜、NDVI、EVI 计算,最后使用质量筛选函数过滤图像,并生成中值合成图像。其中,变量 `sentinel` 是 Sentinel-2 图像集合,通过 `filterDate()`、`filterBounds()` 进行时间和空间筛选;`map()` 函数内的代码则是对每个图像进行云掩膜、NDVI、EVI 计算;`maskClouds()`、`calculateNDVI()`、`calculateEVI()` 分别是云掩膜、NDVI、EVI 计算的函数;`sentinel_ndvi_evi` 变量是应用了云掩膜、NDVI、EVI 计算的图像集合,并选择了所需的波段;`filterQuality()` 是质量筛选函数,根据 NDVI 和 EVI 的质量指标进行筛选;`sentinel_filtered` 是经过质量筛选后的图像集合;`sentinel_median` 是经过中值合成的结果,用于可视化。
Google Earth Engine进行去云处理并逐月计算ndvi并获取时间序列的代码
以下是Google Earth Engine进行去云处理并逐月计算ndvi并获取时间序列的代码:
```javascript
// Load Landsat 8 surface reflectance data.
var collection = ee.ImageCollection('LANDSAT/LC08/C01/T1_SR')
.filterDate('2013-01-01', '2021-12-31')
.filterBounds(geometry)
.map(function(image) {
var qa = image.select('pixel_qa');
// Bits 3 and 5 are cloud and cloud shadow, respectively.
var cloud = qa.bitwiseAnd(1 << 3)
.or(qa.bitwiseAnd(1 << 5));
// Remove cloud and cloud shadow pixels.
var masked = image.updateMask(cloud.not());
// Compute NDVI.
var ndvi = masked.normalizedDifference(['B5', 'B4'])
.rename('NDVI');
// Add time band.
var time = image.metadata('system:time_start')
.divide(1000 * 60 * 60 * 24 * 30)
.floor()
.int()
.rename('time');
return masked.addBands(ndvi).addBands(time);
});
// Create monthly composites and reduce to mean.
var monthlyComposites = ee.ImageCollection.fromImages(
ee.List.sequence(0, 103).map(function(month) {
var filtered = collection.filter(ee.Filter.eq('time', month));
var composite = filtered.mean();
return composite.set('month', month);
})
);
// Get NDVI time series.
var ndviTimeSeries = monthlyComposites.select('NDVI')
.reduceRegions({
collection: geometry,
reducer: ee.Reducer.mean(),
scale: 30
})
.get('groups')
.map(function(group) {
return ee.Feature(geometry)
.set('NDVI', group.mean)
.set('month', group.month);
});
print(ndviTimeSeries);
```
该代码首先加载了Landsat 8表面反射数据,并根据日期和地理位置筛选出需要的数据。然后,代码对每个图像进行去云处理,计算NDVI,并添加时间带。接下来,代码从每个月的图像集合中创建月度组合,并将其减少为平均值。最后,代码从NDVI时间序列中获取数据,并按月份和位置进行分组。最终,代码输出ndviTimeSeries。
阅读全文
相关推荐



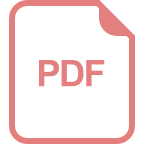











