请用【c++】帮我写一段快排代码,并帮我讲解下实现逻辑
时间: 2024-09-15 22:07:53 浏览: 37
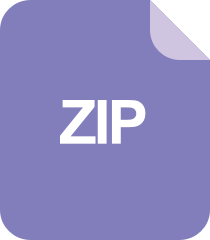
我的世界简易版(C++编写,源代码+程序)已测

当然,这里是一个简单的快速排序算法的C++实现:
```cpp
#include <iostream>
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
int partition (int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high- 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr) / sizeof(arr[0]);
quickSort(arr, 0, n - 1);
std::cout << "Sorted array: \n";
for (int i=0; i < n; i++)
std::cout << arr[i] << " ";
return 0;
}
```
**实现逻辑**:
1. **选择基准元素**:通常选择数组最后一个元素作为基准`pivot`。
2. **分区操作**:从数组的第一个元素开始遍历到倒数第二个元素,如果当前元素小于`pivot`,就将它与`i+1`位置的元素交换(这里`i`用于记录比`pivot`小的元素的下标)。这样,遍历结束后,`pivot`左边的元素都小于`pivot`,右边的元素都大于等于`pivot`。
3. **递归划分**:对基准两侧的子数组分别进行快速排序,直到子数组只剩下一个元素(此时已经有序),递归结束。
4. **主函数**:初始化数组,调用`quickSort`函数对整个数组进行排序。
快速排序是一种分治策略的典型应用,平均时间复杂度为O(n log n),但在最坏的情况下(输入完全逆序),时间复杂度会退化到O(n^2)。
阅读全文
相关推荐
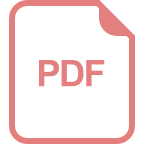
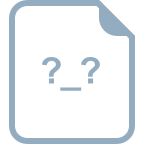













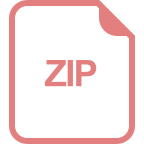
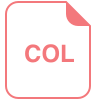
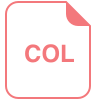