c++用ffmpeg创建rtsp服务器
时间: 2024-06-09 10:07:38 浏览: 26
要在C++中使用FFmpeg创建RTSP服务器,您需要遵循以下步骤:
1. 安装FFmpeg库并将其包含到您的C++项目中。
2. 使用FFmpeg API编写C++代码来处理视频和音频流。
3. 将处理后的视频和音频流发送到RTSP服务器上。
4. 创建一个RTSP服务器并等待客户端连接。
5. 当客户端连接到服务器时,将流数据发送到客户端。
下面是一些示例代码,其中包括使用FFmpeg API来读取视频文件并将其发送到RTSP服务器的示例:
```cpp
// 初始化FFmpeg库
av_register_all();
// 打开视频文件
AVFormatContext* pFormatCtx = NULL;
if (avformat_open_input(&pFormatCtx, "video.mp4", NULL, NULL) != 0) {
// 打开文件失败
return -1;
}
// 获取视频流信息
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
// 获取流信息失败
return -1;
}
// 获取视频流
AVCodec* pCodec = NULL;
AVCodecContext* pCodecCtx = NULL;
int videoStream = -1;
for (int i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStream = i;
pCodecCtx = pFormatCtx->streams[i]->codec;
pCodec = avcodec_find_decoder(pCodecCtx->codec_id);
if (pCodec == NULL) {
// 找不到解码器
return -1;
}
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
// 打开解码器失败
return -1;
}
break;
}
}
// 创建RTSP服务器
AVOutputFormat* pOutputFmt = av_guess_format("rtsp", NULL, NULL);
if (pOutputFmt == NULL) {
// 创建RTSP服务器失败
return -1;
}
AVFormatContext* pOutputCtx = NULL;
if (avformat_alloc_output_context2(&pOutputCtx, pOutputFmt, "rtsp://localhost:8554/test", NULL) < 0) {
// 创建输出格式上下文失败
return -1;
}
AVStream* pStream = avformat_new_stream(pOutputCtx, pCodec);
if (pStream == NULL) {
// 创建流失败
return -1;
}
if (avcodec_parameters_from_context(pStream->codecpar, pCodecCtx) < 0) {
// 复制参数失败
return -1;
}
if (avio_open(&pOutputCtx->pb, "rtsp://localhost:8554/test", AVIO_FLAG_WRITE) < 0) {
// 打开输出流失败
return -1;
}
if (avformat_write_header(pOutputCtx, NULL) < 0) {
// 写入头信息失败
return -1;
}
// 发送视频流到RTSP服务器
AVPacket packet;
while (av_read_frame(pFormatCtx, &packet) >= 0) {
if (packet.stream_index == videoStream) {
av_packet_rescale_ts(&packet, pFormatCtx->streams[videoStream]->time_base, pStream->time_base);
if (av_write_frame(pOutputCtx, &packet) < 0) {
// 发送数据失败
return -1;
}
}
av_packet_unref(&packet);
}
// 关闭RTSP服务器
av_write_trailer(pOutputCtx);
avio_close(pOutputCtx->pb);
avformat_free_context(pOutputCtx);
avformat_close_input(&pFormatCtx);
```
这只是一个简单的示例,您需要根据您的具体需求进行适当的修改。同时,您还需要实现RTSP服务器的客户端连接和流数据发送等功能。
相关推荐
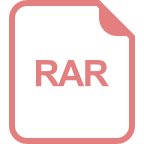
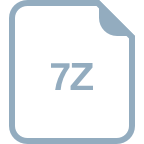
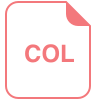
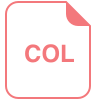
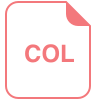
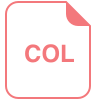
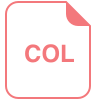






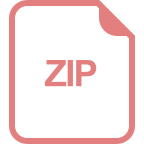
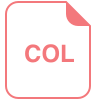