写一个unity的无限滚动列表
时间: 2023-05-28 09:02:27 浏览: 303
Unity的无限滚动列表通常使用ScrollRect组件来实现。以下是一个基本的步骤:
1.在场景中创建一个ScrollView,给ScrollView添加ScrollRect组件;
2.在ScrollView中创建一个Viewport,设置Viewport的Anchor为Stretch,设置Pivot为(0,1),设置Width和Height为滚动列表中的一项的大小;
3.在Viewport中创建一个Content,设置Content的Anchor为TopLeft,设置Pivot为(0,1),设置宽度为Viewport的宽度,高度为所有项的高度之和;
4.在Content中创建多个子对象,每个子对象代表列表中的一项;
5.为每个子对象添加一个LayoutElement组件,设置该组件的Preferred Height和Min Height为该项的高度;
6.编写脚本进行列表的控制,脚本需要继承自MonoBehaviour,并包含以下变量和方法:
```
[SerializeField] private GameObject listItemPrefab; // 列表项的模版
[SerializeField] private int totalItemCount; // 列表项的总数
[SerializeField] private float listItemHeight; // 列表项的高度
[SerializeField] private Transform content; // Content对象
[SerializeField] private List<GameObject> spawnedItems; // 已经生成的列表项
[SerializeField] private float spawnYPosition = 0f; // 第一个生成的列表项的y坐标
[SerializeField] private float destroyYPosition = -100f; // 超出该y坐标的列表项将被销毁
private Vector2 lastContentPos; // 上一帧Content的位置
private void Awake()
{
spawnedItems = new List<GameObject>();
SpawnItems();
}
private void Update()
{
UpdateVisibleItems();
}
private void SpawnItems()
{
float spawnXPosition = 0f;
for (int i = 0; i < totalItemCount; i++)
{
GameObject spawnedItem = Instantiate(listItemPrefab, content);
spawnedItem.transform.localPosition = new Vector2(spawnXPosition, spawnYPosition);
spawnedItem.GetComponentInChildren<Text>().text = "Item " + i;
spawnYPosition -= listItemHeight;
spawnedItems.Add(spawnedItem);
}
}
private void UpdateVisibleItems()
{
Vector2 contentPos = content.anchoredPosition;
if (contentPos != lastContentPos)
{
float contentTopY = contentPos.y + content.rect.yMin;
float contentBottomY = contentPos.y + content.rect.yMax;
for (int i = 0; i < spawnedItems.Count; i++)
{
float itemY = spawnedItems[i].GetComponent<RectTransform>().anchoredPosition.y;
if (itemY + listItemHeight < contentTopY || itemY > contentBottomY)
{
spawnedItems[i].SetActive(false);
}
else
{
spawnedItems[i].SetActive(true);
}
if (itemY < destroyYPosition && spawnedItems[i].activeSelf)
{
spawnedItems[i].SetActive(false);
}
}
lastContentPos = contentPos;
}
}
```
7.在脚本中添加SpawnItems和UpdateVisibleItems方法,SpawnItems用于生成所有的列表项,UpdateVisibleItems用于更新当前在ScrollView中可见的列表项;
以上就是一个基本的Unity无限滚动列表的实现,可以根据实际情况进行修改和扩展。
相关推荐
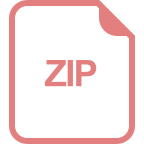
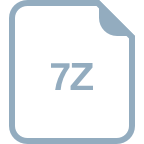














