unity无限滚动条脚本
时间: 2023-06-28 08:15:25 浏览: 47
实现无限滚动条的方法通常是在滚动到某个阈值后,将当前显示的内容复制一份添加到滚动列表的末尾,并将滚动位置重置到起始位置。以下是一个示例脚本可以实现这个功能:
```csharp
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class InfiniteScroll : MonoBehaviour
{
public RectTransform content;
public RectTransform prefab;
public int itemCount = 10;
public float itemSize = 100f;
public float spacing = 10f;
private List<RectTransform> items = new List<RectTransform>();
private float contentSize;
private int currentIndex = 0;
private void Start()
{
contentSize = (itemSize + spacing) * itemCount;
for (int i = 0; i < itemCount; i++)
{
RectTransform item = Instantiate(prefab);
item.SetParent(content);
item.localScale = Vector3.one;
item.anchoredPosition = new Vector2(0, -i * (itemSize + spacing));
items.Add(item);
}
}
private void Update()
{
if (content.anchoredPosition.y < -currentIndex * (itemSize + spacing))
{
currentIndex--;
if (currentIndex < 0)
{
currentIndex = itemCount - 1;
}
RectTransform item = items[currentIndex];
item.anchoredPosition = new Vector2(0, contentSize - (itemSize + spacing) * currentIndex);
items.Remove(item);
items.Insert(0, item);
}
if (content.anchoredPosition.y > -(currentIndex + 1) * (itemSize + spacing))
{
currentIndex++;
if (currentIndex >= itemCount)
{
currentIndex = 0;
}
RectTransform item = items[items.Count - 1];
item.anchoredPosition = new Vector2(0, -currentIndex * (itemSize + spacing));
items.Remove(item);
items.Add(item);
}
}
}
```
这个脚本会在Start函数中创建初始的滚动列表,并在Update函数中检查滚动位置是否超出当前显示的内容。如果滚动位置超出当前显示的内容,它会将当前显示的内容复制一份添加到滚动列表的末尾,并将滚动位置重置到起始位置。这个脚本需要一个包含一个滚动条的RectTransform作为content变量的引用,以及一个包含一个填充项的RectTransform作为prefab变量的引用。你可以调整itemCount、itemSize和spacing变量来控制滚动条中显示的内容。
相关推荐
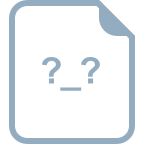
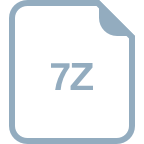














