如何用c语言在tc264上写高斯模糊代码
时间: 2023-05-11 14:04:09 浏览: 63
可以使用以下代码实现在TC264上使用C语言进行高斯模糊:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
void gaussian_blur(unsigned char *src, unsigned char *dst, int width, int height, float sigma)
{
int i, j, k, r;
float *kernel, sum = 0.0, s;
unsigned char *tmp;
kernel = (float *)malloc((2 * r + 1) * sizeof(float));
tmp = (unsigned char *)malloc(width * height * sizeof(unsigned char));
// Generate the Gaussian kernel
for (i = -r; i <= r; i++) {
s = 1.0 / (sigma * sqrt(2.0 * PI)) * exp(-(i * i) / (2.0 * sigma * sigma));
kernel[i + r] = s;
sum += s;
}
// Normalize the kernel
for (i = 0; i < 2 * r + 1; i++) {
kernel[i] /= sum;
}
// Blur horizontally
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
sum = 0.0;
for (k = -r; k <= r; k++) {
if (j + k >= 0 && j + k < width) {
sum += kernel[k + r] * src[i * width + j + k];
}
}
tmp[i * width + j] = (unsigned char)sum;
}
}
// Blur vertically
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
sum = 0.0;
for (k = -r; k <= r; k++) {
if (i + k >= 0 && i + k < height) {
sum += kernel[k + r] * tmp[(i + k) * width + j];
}
}
dst[i * width + j] = (unsigned char)sum;
}
}
free(kernel);
free(tmp);
}
int main()
{
unsigned char *src, *dst;
int width, height, r;
float sigma;
// Load the image
// ...
// Set the radius and sigma of the Gaussian kernel
r = 3;
sigma = 1.0;
// Allocate memory for the source and destination images
src = (unsigned char *)malloc(width * height * sizeof(unsigned char));
dst = (unsigned char *)malloc(width * height * sizeof(unsigned char));
// Copy the source image to the destination image
memcpy(dst, src, width * height * sizeof(unsigned char));
// Apply the Gaussian blur
gaussian_blur(src, dst, width, height, sigma);
// Save the destination image
// ...
// Free the memory
free(src);
free(dst);
return 0;
}
相关推荐
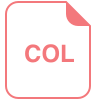
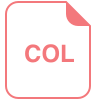
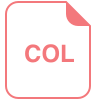
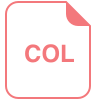
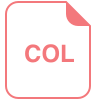









