用C语言写高斯模糊代码
时间: 2023-05-28 22:06:44 浏览: 122
以下是使用C语言实现高斯模糊的代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
#define STB_IMAGE_WRITE_IMPLEMENTATION
#include "stb_image_write.h"
#define PI 3.14159265358979323846
//高斯分布的计算函数
float gaussian(float x, float sigma) {
return exp(-x*x / (2*sigma*sigma)) / (sqrt(2*PI)*sigma);
}
//高斯模糊函数
void gaussian_blur(unsigned char *image, int width, int height, int channels, float sigma) {
int radius = (int)(3*sigma); //高斯核半径
int size = 2*radius + 1; //高斯核大小
float *kernel = (float*)malloc(size * sizeof(float)); //高斯核数组
float sum = 0; //高斯核元素和
//计算高斯核数组
for (int i = 0; i < size; i++) {
kernel[i] = gaussian(i - radius, sigma);
sum += kernel[i];
}
//归一化高斯核数组
for (int i = 0; i < size; i++) {
kernel[i] /= sum;
}
//逐行逐列进行高斯模糊
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
for (int c = 0; c < channels; c++) {
float sum = 0;
//遍历高斯核
for (int i = -radius; i <= radius; i++) {
//边缘像素处理:超出图像范围的像素值为0
int px = x + i;
if (px < 0) px = 0;
else if (px >= width) px = width - 1;
//计算高斯核权重,并累加乘积
float weight = kernel[i+radius];
sum += weight * image[(y*width + px)*channels + c];
}
//将乘积结果写入当前像素值
image[(y*width + x)*channels + c] = (unsigned char)sum;
}
}
}
free(kernel);
}
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s input_image [output_image] [sigma]\n", argv[0]);
return 0;
}
char *input_filename = argv[1];
char *output_filename = argc >= 3 ? argv[2] : "output.jpg";
float sigma = argc >= 4 ? atof(argv[3]) : 1.0f;
int width, height, channels;
unsigned char *image = stbi_load(input_filename, &width, &height, &channels, 0);
if (image == NULL) {
printf("Error loading image %s\n", input_filename);
return 1;
}
gaussian_blur(image, width, height, channels, sigma);
stbi_write_jpg(output_filename, width, height, channels, image, 100);
stbi_image_free(image);
return 0;
}
阅读全文
相关推荐



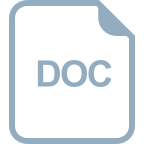
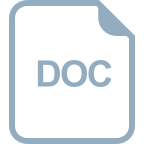
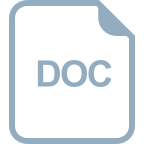
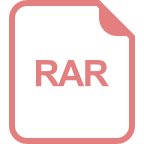
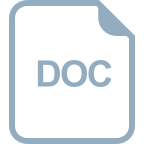
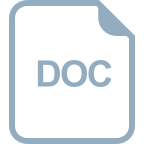
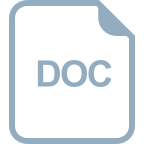


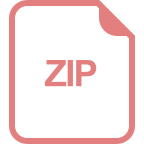
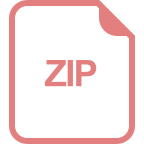
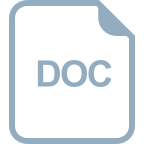
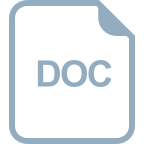