1 #include <sys/types.h> 2 #include <unistd.h> 3 #include <stdio.h> 4 #include <stdlib.h> 5 int main() 6 { 7 pid_t pid; 8 char *message; 9 int n = 3; 10 11 printf("fork program starting\n"); 12 pid = fork(); 13 switch(pid) 14 { 15 case -1: 16 perror("fork failed"); 17 exit(1); 18 case 0: 19 message = "This is the child"; 20 n = 4; 21 break; 22 default: 23 message = "This is the parent"; 24 n--; 25 break; 26 } 27 28 for(; n > 0; n--) { 29 puts(message); 30 sleep(1); 31 } 32 33 exit(0); 34 }
时间: 2024-02-10 11:33:49 浏览: 82
这是一个使用fork()函数创建子进程的简单程序。在程序开始处,会输出一行提示信息。然后通过调用fork()函数创建一个子进程,fork()函数会返回一个pid值,通过这个pid值可以判断当前是在父进程还是在子进程中执行代码。如果pid值为-1,则表示创建子进程失败,程序将输出错误信息并退出。如果pid值为0,则表示当前正在子进程中执行,程序会将message变量的值设置为"This is the child",并将n的值设置为4。否则,表示当前正在父进程中执行,程序会将message变量的值设置为"This is the parent",并将n的值减1。最后,程序会通过循环输出message变量的值,每次输出后休眠1秒钟,循环结束后退出程序。
相关问题
给出如下C程序,文件名为ex_fork.c,请分析在Linux下程序运行的结果,并说明原因。 1 #include <sys/types.h> 2 #include <unistd.h> 3 #include <stdio.h> 4 #include <stdlib.h> 5 int main() 6 { 7 pid_t pid; 8 char *message; 9 int n = 3; 10 11 printf("fork program starting "); 12 pid = fork(); 13 switch(pid) 14 { 15 case -1: 16 perror("fork failed"); 17 exit(1); 18 case 0: 19 message = "This is the child"; 20 n = 4; 21 break; 22 default: 23 message = "This is the parent"; 24 n++; 25 break; 26 } 27 28 for(; n > 0; n--) { 29 puts(message); 30 sleep(1); 31 } 32 33 exit(0); 34 }
该程序的运行结果是创建一个子进程,父进程和子进程分别输出自己的信息,并交替输出若干次后退出。
具体来说,程序运行后,先输出一条提示信息"fork program starting",然后调用fork()函数创建一个子进程。fork()函数返回值有三种情况:
- 返回-1,代表fork()调用失败;
- 返回0,代表当前进程为子进程;
- 返回一个大于0的进程ID,代表当前进程为父进程,返回的进程ID是子进程的进程ID。
在父进程中,pid的值为子进程的进程ID,而在子进程中,pid的值为0。根据pid的值,程序使用switch语句进行分支处理:
- 如果pid为-1,表示fork()调用失败,程序将输出错误信息并退出;
- 如果pid为0,表示当前进程为子进程,此时将message指向"This is the child"字符串,并将n的值修改为4;
- 如果pid大于0,表示当前进程为父进程,此时将message指向"This is the parent"字符串,并将n的值加1。
接下来,程序通过for循环输出message的值,输出次数为n的值。由于父进程中n的值为4,而子进程中n的值为3,因此父进程输出5次,而子进程输出4次。在每次输出后,程序通过sleep(1)函数使当前进程暂停1秒钟,然后再进行下一次输出。最后,程序调用exit(0)函数退出进程。
因此,程序最终的输出结果类似于下面这样:
```
fork program starting This is the parent
This is the parent
This is the child
This is the parent
This is the child
This is the parent
This is the child
This is the parent
```
#include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #include <unistd.h> #include <fcntl.h> #include <pthread.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <ctype.h> #include <sys/pps.h> #include <sys/types.h> #include "camera.h" #include <encoder.h> #include "ppsFunc.h" #include "timeDiff.h"
这是一段C语言的代码,使用了一些系统库和自定义头文件。其中包括了网络编程相关的库,如sys/socket.h和netinet/in.h,以及多线程相关的库pthread.h。另外还有一些自定义的头文件,如camera.h和encoder.h,可能是该程序所需的一些外部依赖。该程序可能是一个视频流的采集和编码程序,同时还使用了PPS和时间差等功能。
阅读全文
相关推荐
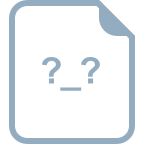
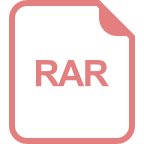










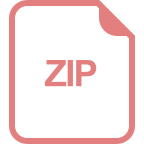
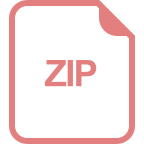
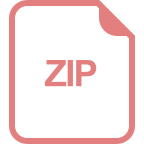
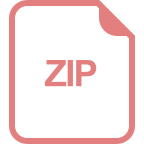