include <unistd.h> #include <stdio.h> #include <stdlib.h> #include <sys/types.h> #include <sys/wait.h> int main(void){ pid_t a; a = fork(); if (a < 0) { perror("fork failed"); exit(1); } if (a == 0) { execlp ("ps" ,"ps",NULL); execlp ("ls" ,"ls","-al","/etc/passwd ",NULL); return 1; } else { wait(NULL); printf("father exit\n");} return 0; }改正以上代码
时间: 2024-02-14 15:16:28 浏览: 83
根据你提供的代码,我们可以看出,子进程中有两个 `execlp` 函数调用,这意味着子进程将执行两个程序,而不是一个程序。此外,在第二个 `execlp` 函数调用中,你在 `/etc/passwd` 后面添加了一个空格,这可能会导致程序无法正常运行。
下面是改正后的代码:
```c
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
int main(void) {
pid_t pid;
pid = fork();
if (pid < 0) {
perror("fork failed");
exit(1);
} else if (pid == 0) {
execlp("ps", "ps", NULL);
perror("execlp failed");
exit(1);
} else {
wait(NULL);
printf("child process finished\n");
}
return 0;
}
```
在上面的代码中,我们只调用了一个 `execlp` 函数,并且没有在 `/etc/passwd` 后面添加空格。如果 `execlp` 调用失败,我们使用 `perror` 函数输出错误信息。此外,我们还将 `printf` 语句修改为 "child process finished",以更清晰地表达程序的意图。
阅读全文
相关推荐
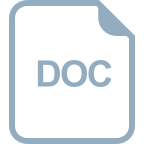
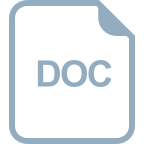
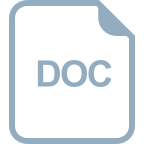
















