用Java实现addition()方法将传入变量a和变量n。 sum=a+aa+aaa+aaaa+aa...a (n个a),给定a和n的值,要求计算得到sum的值。
时间: 2024-09-20 16:03:25 浏览: 84
在Java中,你可以创建一个名为`SumOfAverages`的类,其中包含一个静态方法`addition(int a, int n)`,用于计算给定a和n的情况下,连续相加a的n次幂的和。这里是一个简单的实现:
```java
public class SumOfAverages {
public static int addition(int a, int n) {
int sum = 0;
for (int i = 0; i < n; i++) {
// 对a进行i次乘法然后加到总和上
sum += Math.pow(a, i + 1);
}
return sum;
}
public static void main(String[] args) {
int a = 2; // 你想要相加的数字
int n = 5; // 相加次数
int result = addition(a, n);
System.out.println("The sum is: " + result);
}
}
```
在这个例子中,`Math.pow(a, i + 1)`计算了a的(i+1)次方,然后将其累加到`sum`中。当你运行这个程序并传入具体的a和n值时,它会返回相应的和。
相关问题
Write+code+for+a+function+with+the+following+prototype: /*+Addition+that+saturates+to+TMin+or+TMax+*
Sure! Here's an implementation of the function you requested:
```c++
#include <limits.h>
int saturating_add(int x, int y) {
int sum = x + y;
int neg_over = x < 0 && y < 0 && sum >= 0;
int pos_over = x >= 0 && y >= 0 && sum < 0;
int is_overflow = neg_over || pos_over;
int tmin = INT_MIN;
int tmax = INT_MAX;
int result = is_overflow ? (sum < 0 ? tmin : tmax) : sum;
return result;
}
```
This function takes two integer arguments `x` and `y`, and returns their sum. However, if the sum overflows the range of representable integers, the function "saturates" the result to either the minimum or maximum representable integer value, depending on the sign of the operands.
To implement this behavior, we first compute the sum of `x` and `y`. We then check whether the sum overflows by comparing the signs of `x`, `y`, and `sum`. If `x` and `y` have the same sign and `sum` has the opposite sign, then overflow has occurred. We store this information in the `is_overflow` variable.
If overflow has occurred, we set the result to either the minimum or maximum representable integer value, depending on whether the sum is negative or positive. Otherwise, we set the result to the sum itself.
I hope this helps! Let me know if you have any further questions.
public class Addition { // 无参构造方法 public Addition(){} // add方法,计算int类型相加的结果 public int add(int a, int b){ return a + b; } // add方法,计算long类型相加的结果 public long add(long a, long b){ return a + b; } // add方法,计算double类型相加的结果 public double add(double a, double b){ return a + b; } // add方法,计算float类型相加的结果 public float add(float a, float b){ return a + b; } // main方法 public static void main(String[] args){ Addition addition = new Addition(); int result1 = addition.add(1, 2); long result2 = addition.add(2L, 3L); double result3 = addition.add(3.0, 4.0); float result4 = addition.add(4.0f, 5.0f); System.out.println("1 + 2 = " + result1); System.out.println("2L + 3L = " + result2); System.out.println("3.0 + 4.0 = " + result3); System.out.println("4.0f + 5.0f = " + result4); } }
这段代码定义了一个名为 Addition 的类,其中包含了四个重载的 add 方法,分别用于计算 int、long、double、float 类型的加法运算。在 main 方法中,我们分别创建了 Addition 类的对象 addition,并调用了四个不同类型的 add 方法,将结果保存在不同类型的变量中,并使用 System.out.println 方法输出了这些结果。最终结果应该是:1 + 2 = 3,2L + 3L = 5,3.0 + 4.0 = 7.0,4.0f + 5.0f = 9.0。
阅读全文
相关推荐
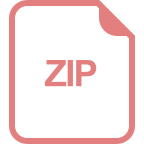
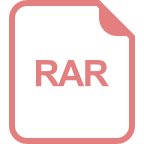
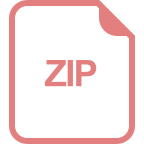
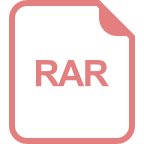
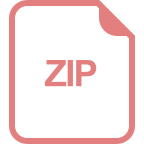
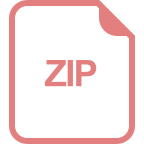
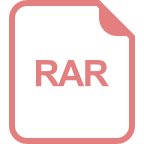
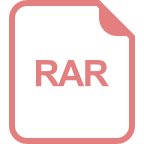
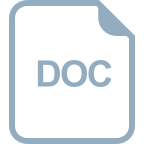







