用c++ opencv写一个基于SIFT算法的局部特征匹配
时间: 2024-02-23 15:01:40 浏览: 61
好的,下面是一个基于 SIFT 算法的局部特征匹配的 C++ OpenCV 实现。
首先,我们需要导入必要的头文件和命名空间:
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
```
然后,我们可以定义一个函数来执行 SIFT 特征提取和匹配:
```cpp
void siftMatch(const Mat &img1, const Mat &img2, std::vector<Point2f> &pts1, std::vector<Point2f> &pts2)
{
// 创建 SIFT 特征点检测器和描述符提取器
Ptr<Feature2D> detector = SIFT::create();
// 检测关键点,并计算描述符
std::vector<KeyPoint> keypoints1, keypoints2;
Mat descriptors1, descriptors2;
detector->detectAndCompute(img1, Mat(), keypoints1, descriptors1);
detector->detectAndCompute(img2, Mat(), keypoints2, descriptors2);
// 创建暴力匹配器
BFMatcher matcher(NORM_L2);
// 匹配描述符
std::vector<DMatch> matches;
matcher.match(descriptors1, descriptors2, matches);
// 选择最佳匹配
double minDist = 100.0;
for (int i = 0; i < matches.size(); i++)
{
if (matches[i].distance < minDist)
{
minDist = matches[i].distance;
}
}
// 选取最佳匹配的点对
for (int i = 0; i < matches.size(); i++)
{
if (matches[i].distance < 3 * minDist)
{
pts1.push_back(keypoints1[matches[i].queryIdx].pt);
pts2.push_back(keypoints2[matches[i].trainIdx].pt);
}
}
}
```
在这个函数中,我们首先创建了 SIFT 特征点检测器和描述符提取器,然后使用它们检测关键点并计算描述符。接下来,我们创建了一个暴力匹配器,并使用它来匹配描述符。然后我们选择最佳匹配,并选取最佳匹配的点对。
最后,我们可以在主函数中调用这个函数,如下所示:
```cpp
int main()
{
// 读取图像
Mat img1 = imread("img1.jpg");
Mat img2 = imread("img2.jpg");
// 提取关键点并匹配
std::vector<Point2f> pts1, pts2;
siftMatch(img1, img2, pts1, pts2);
// 绘制匹配结果
Mat imgMatches;
drawMatches(img1, keypoints1, img2, keypoints2, matches, imgMatches);
imshow("Matches", imgMatches);
waitKey(0);
return 0;
}
```
在这个示例中,我们首先读取了两张图像。然后,我们调用 `siftMatch` 函数来提取关键点并匹配。最后,我们使用 OpenCV 的 `drawMatches` 函数来绘制匹配结果,并显示在窗口中。
注意,在实际使用中,我们可能需要对提取的关键点进行筛选和优化,以提高匹配的准确性。
阅读全文
相关推荐
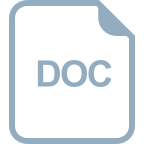
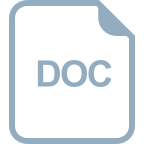
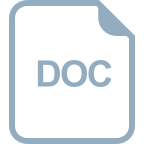

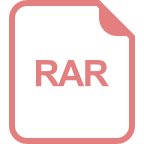
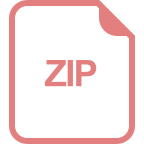
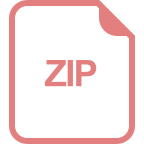
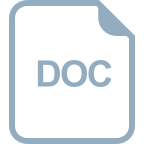







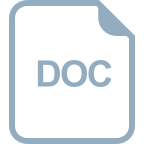