用c#做一个窗体应用程序,实现对数据库的增删查改功能
时间: 2023-06-25 11:04:24 浏览: 112
好的,下面是一个简单的例子,假设你已经有了一个名为“test”的数据库,其中包含一个名为“user”的表,表中包含id、name和age三个字段。
首先,你需要在C#中引用System.Data.SqlClient命名空间。代码如下:
```
using System.Data.SqlClient;
```
然后,你需要定义与数据库连接相关的变量。代码如下:
```
private const string connString = "Data Source=.;Initial Catalog=test;Integrated Security=True";
private SqlConnection conn = new SqlConnection(connString);
private SqlCommand cmd = new SqlCommand();
```
其中,connString是连接字符串,指定了连接的数据库名称、服务器地址以及验证方式。这里使用Windows身份验证。
接着,你可以定义增删查改操作相关的函数。代码如下:
```
// 查询所有用户
private void QueryAllUsers()
{
try
{
conn.Open();
cmd.Connection = conn;
cmd.CommandText = "SELECT * FROM user";
SqlDataReader reader = cmd.ExecuteReader();
while (reader.Read())
{
string id = reader["id"].ToString();
string name = reader["name"].ToString();
string age = reader["age"].ToString();
// 在界面上展示用户信息
// ...
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
conn.Close();
}
}
// 根据ID查询用户
private void QueryUserById(string id)
{
try
{
conn.Open();
cmd.Connection = conn;
cmd.CommandText = $"SELECT * FROM user WHERE id='{id}'";
SqlDataReader reader = cmd.ExecuteReader();
if (reader.Read())
{
string name = reader["name"].ToString();
string age = reader["age"].ToString();
// 在界面上展示用户信息
// ...
}
else
{
MessageBox.Show("用户不存在");
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
conn.Close();
}
}
// 添加用户
private void AddUser(string name, string age)
{
try
{
conn.Open();
cmd.Connection = conn;
cmd.CommandText = $"INSERT INTO user (name, age) VALUES ('{name}', {age})";
int result = cmd.ExecuteNonQuery();
if (result > 0)
{
MessageBox.Show("添加成功");
}
else
{
MessageBox.Show("添加失败");
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
conn.Close();
}
}
// 修改用户
private void UpdateUser(string id, string name, string age)
{
try
{
conn.Open();
cmd.Connection = conn;
cmd.CommandText = $"UPDATE user SET name='{name}', age={age} WHERE id='{id}'";
int result = cmd.ExecuteNonQuery();
if (result > 0)
{
MessageBox.Show("修改成功");
}
else
{
MessageBox.Show("修改失败");
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
conn.Close();
}
}
// 删除用户
private void DeleteUser(string id)
{
try
{
conn.Open();
cmd.Connection = conn;
cmd.CommandText = $"DELETE FROM user WHERE id='{id}'";
int result = cmd.ExecuteNonQuery();
if (result > 0)
{
MessageBox.Show("删除成功");
}
else
{
MessageBox.Show("删除失败");
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
conn.Close();
}
}
```
最后,你需要在窗体中添加相应的控件和事件处理函数,实现对数据库的操作。代码如下:
```
private void btnQueryAll_Click(object sender, EventArgs e)
{
QueryAllUsers();
}
private void btnQueryById_Click(object sender, EventArgs e)
{
string id = txtId.Text.Trim();
QueryUserById(id);
}
private void btnAdd_Click(object sender, EventArgs e)
{
string name = txtName.Text.Trim();
string age = txtAge.Text.Trim();
AddUser(name, age);
}
private void btnUpdate_Click(object sender, EventArgs e)
{
string id = txtId.Text.Trim();
string name = txtName.Text.Trim();
string age = txtAge.Text.Trim();
UpdateUser(id, name, age);
}
private void btnDelete_Click(object sender, EventArgs e)
{
string id = txtId.Text.Trim();
DeleteUser(id);
}
```
以上是一个简单的窗体应用程序,实现了对数据库的增删查改功能。当然,为了保证程序的安全性和性能,你还需要进行更全面的异常处理、参数验证以及SQL注入等方面的防范。
阅读全文
相关推荐















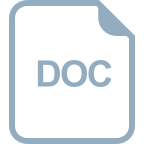

